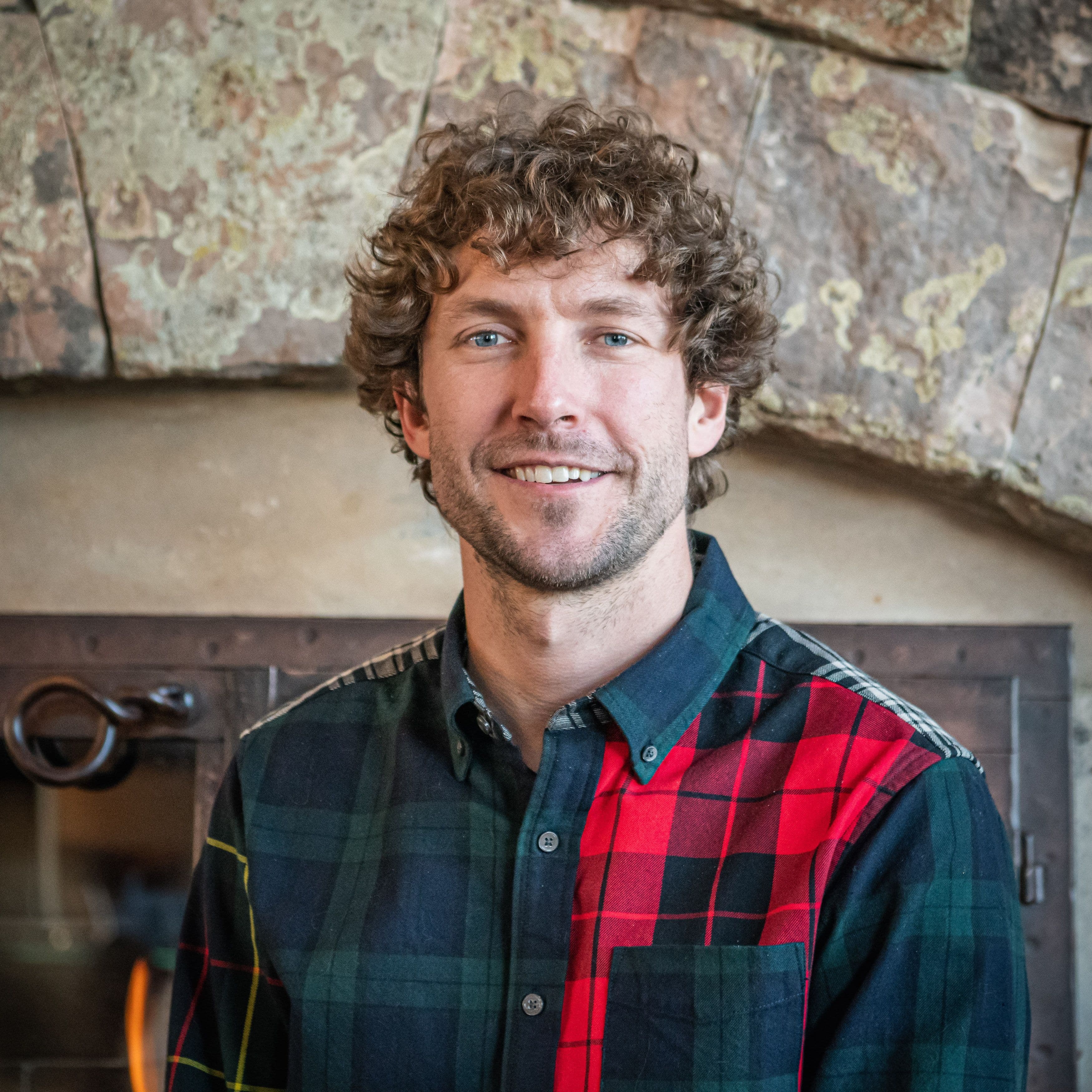
Set up ESLint for best practices
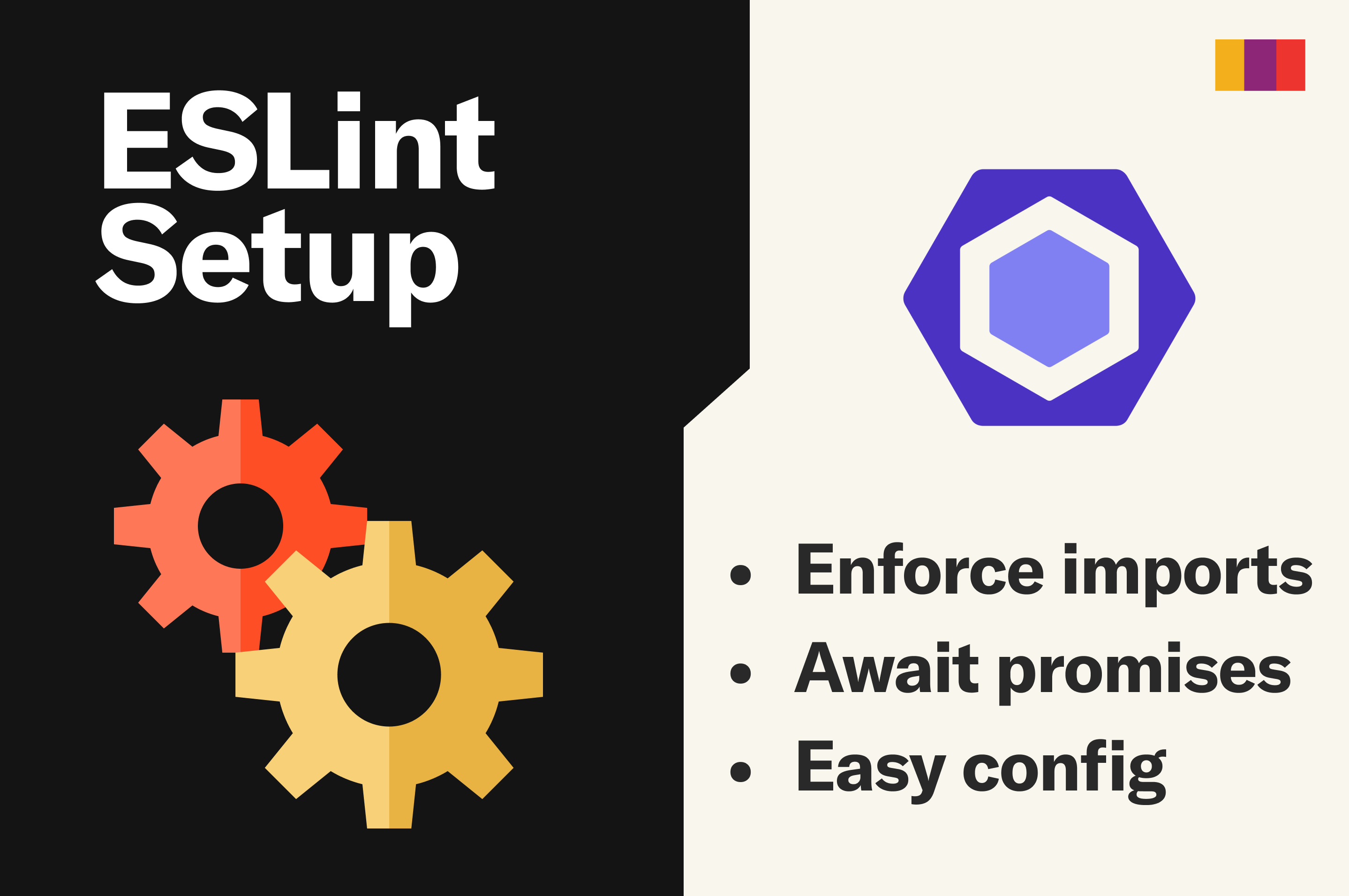
ESLint is a linter for JavaScript / TypeScript that goes beyond type checking to enforce best practices. For instance, ensuring you don’t have unused parameters (which may indicate a bug where you shadowed a variable or don’t await a promise. The tool is powerful and allows extensions and plugins, such as React-specific rules.
This post looks at configuring ESLint for best practices for serverless functions, Convex in particular.
Configuring ESLint
You can start from scratch with npm init @eslint/config
and hand-craft your own file, or use an opinionated collection of best practices from a package like Airbnb’s eslint-config-airbnb
.
See below for a configuration that includes my preferred defaults, including Convex best practices.
You can add a package.json script to allow you to do npm run lint
, like:
1"scripts": {
2 "lint": "eslint . --ext .ts,.tsx",
3
Awaiting all promises in server functions
If you are running code on the server and you start an async function, it’s best practice to await its result before returning a result to the client. Having code run after a function returns is best pushed into a function that’s explicitly run asynchronously, like Convex’s scheduled functions.
In Convex, all database operations like db.get
, db.query
, db.delete
etc. are async functions. In addition, operations in an action, like ctx.scheduler.*
need to be awaited. Otherwise it may or may not actually run, depending when your function returns. Many serverless providers have this gotcha. Convex will print a helpful error message to your logs when you do this, but ESLint can help you catch it earlier. Just add this rule in the rules
section:
1"@typescript-eslint/no-floating-promises": "error",
2
This requires "@typescript-eslint"
listed in the config’s "plugins"
, which comes from the @typescript-eslint/eslint-plugin@latest
npm package.
Enforcing imports with ESLint
When you want to extend the behavior of functions that come from an npm package or codegen, one way of building abstractions is to wrap the function call with a utility function. This way you can add behavior onto the original function. For instance, if you’re using a library like Ents with Convex, you want developers to interact with the database through the Ent table
functions instead of ctx.db
directly, as they can have side-effects like cascading deletes. Read more about Ent custom function wrapping here.
Another common use case is for the custom functions pattern. Custom functions let you define a new version of a server function, like query, mutation, or action that do some common work before a request. This pattern is opt-in, so if you’re doing special authorization checks for a custom query
, another developer can still import the “raw” query
function from the convex/_generated/server
codegen file. To enforce the usage of your custom function, you can use an ESLint rule: no-restricted-imports
.
no-restricted-imports
To disallow importing the wrapped functions via ESLint, use no-restricted-imports to define this rule in the rules
section:
1"no-restricted-imports": [
2 "error",
3 {
4 patterns: [
5 {
6 group: ["*/_generated/server"],
7 importNames: ["query", "mutation", "action"],
8 message: "Use functions.ts for query, mutation, or action",
9 },
10 ],
11 },
12],
13
This throws an error whenever someone imports query
, mutation
, or action
from anything ending in _generated/server
. Instead, they are encouraged to use the functions.ts
version of them.
Note: you may also want to include internalQuery
, internalMutation
, and internalAction
to also discourage importing these raw functions. Inner here means they can only be called from other server functions. For the above example, I only care about enforcing usage of custom functions for client-facing APIs.
convex/functions.ts
In convex/functions.ts
, I define custom functions that ensure all queries, mutations, and actions are only run by users from my company, by looking at the verfied email’s domain:
1/* eslint-disable no-restricted-imports */
2import {
3 action as actionRaw,
4 mutation as mutationRaw,
5 query as queryRaw,
6} from "./_generated/server";
7/* eslint-enable no-restricted-imports */
8import { ConvexError } from "convex/values";
9import { customAction, customCtx, customMutation, customQuery } from "convex-helpers/server/customFunctions";
10
11const authCheck = customCtx(async (ctx) => {
12 const identity = await ctx.auth.getUserIdentity();
13 if (!identity) throw new ConvexError("Not authenticated");
14 if (!identity.emailVerified) throw new ConvexError("Email not verified");
15 if (!identity.email?.endsWith("@convex.dev"))
16 throw new ConvexError("Convex emails only");
17 return {};
18});
19
20export const query = customQuery(queryRaw, authCheck);
21export const mutation = customMutation(mutationRaw, authCheck);
22export const action = customAction(actionRaw, authCheck);
23
This exports new query
, mutation
, and action
functions that behave like the ones imported from ./_generated/server
, with an auth check before they run. Now, other files can import them like import { query, mutation } from "./functions";
and use them in place of the originals.
Escape hatch
Note that we’re disabling the no-restricted-imports
rule during the import:
1/* eslint-disable no-restricted-imports */
2import {
3 action as actionRaw,
4 mutation as mutationRaw,
5 query as queryRaw,
6} from "./_generated/server";
7/* eslint-enable no-restricted-imports */
8
We want to limit that import everywhere except this file where we’re wrapping them. This is also how you can get around the rule if you want to use the raw functions somewhere, though I’d encourage you to instead re-export them from functions.ts
like:
1export {
2 queryRaw as publicQuery,
3 mutationRaw as publicMutation,
4 actionRaw as publicAction,
5};
6
Then you explicitly use them as publicQuery
where you want to, and don’t need to make exceptions to ESLint rules more than absolutely necessary.
Full .eslintrc.cjs
This is the configuration I’m using in a React / Vite project to make a CMS on top of Convex:
1module.exports = {
2 env: {
3 browser: true,
4 es2021: true,
5 node: true,
6 },
7 extends: [
8 "eslint:recommended",
9 "plugin:@typescript-eslint/recommended",
10 "plugin:react/recommended",
11 ],
12 ignorePatterns: [".eslintrc.cjs", "convex/_generated", "node_modules"],
13 overrides: [
14 {
15 env: {
16 node: true,
17 },
18 files: [".eslintrc.{js,cjs}"],
19 parserOptions: {
20 sourceType: "script",
21 },
22 },
23 ],
24 parser: "@typescript-eslint/parser",
25 parserOptions: {
26 ecmaVersion: "latest",
27 project: true,
28 sourceType: "module",
29 },
30 plugins: ["@typescript-eslint", "react"],
31 rules: {
32 // Only warn on unused variables, and ignore variables starting with `_`
33 "@typescript-eslint/no-unused-vars": [
34 "warn",
35 { varsIgnorePattern: "^_", argsIgnorePattern: "^_" },
36 ],
37
38 // Await your promises
39 "@typescript-eslint/no-floating-promises": "error",
40
41 // Allow explicit `any`s
42 "@typescript-eslint/no-explicit-any": "off",
43
44 "react/react-in-jsx-scope": "off",
45 "react/prop-types": "off",
46
47 // http://eslint.org/docs/rules/no-restricted-imports
48 "no-restricted-imports": [
49 "error",
50 {
51 patterns: [
52 {
53 group: ["*/_generated/server"],
54 importNames: ["query", "mutation", "action"],
55 message: "Use functions.ts for query, mutation, action",
56 },
57 ],
58 },
59 ],
60 },
61 settings: {
62 react: {
63 version: "detect",
64 },
65 },
66};
67
68
To use this, you’ll need to install these packages:
1npm i -D eslint@latest @typescript-eslint/parser@latest @typescript-eslint/eslint-plugin@latest eslint-plugin-react@latest
2
"plugins", "extends", and configurations
For those curious, the difference between “plugins” and “extends” in the config is that a plugin doesn’t add any rules by default. It includes rules you can explicitly use, as well as rule configurations you can add in the “extends” section which adds many rules at once. For instance, plugin:react/recommended
in the extends
section comes from the react
plugin in the plugins
list, which comes from the eslint-plugin-react@latest
npm package. Some packages don’t provide a plugin and only provide a configuration to use in “extends” and are named as such, like eslint-config-airbnb
.
Summary
We looked at adding ESLint to your project and special rules to enforce best practices in Convex. This is just one of the ways to make your app more secure. To see other best practices, check out other Patterns posts.
Convex is the backend platform with everything you need to build your full-stack AI project. Cloud functions, a database, file storage, scheduling, workflow, vector search, and realtime updates fit together seamlessly.