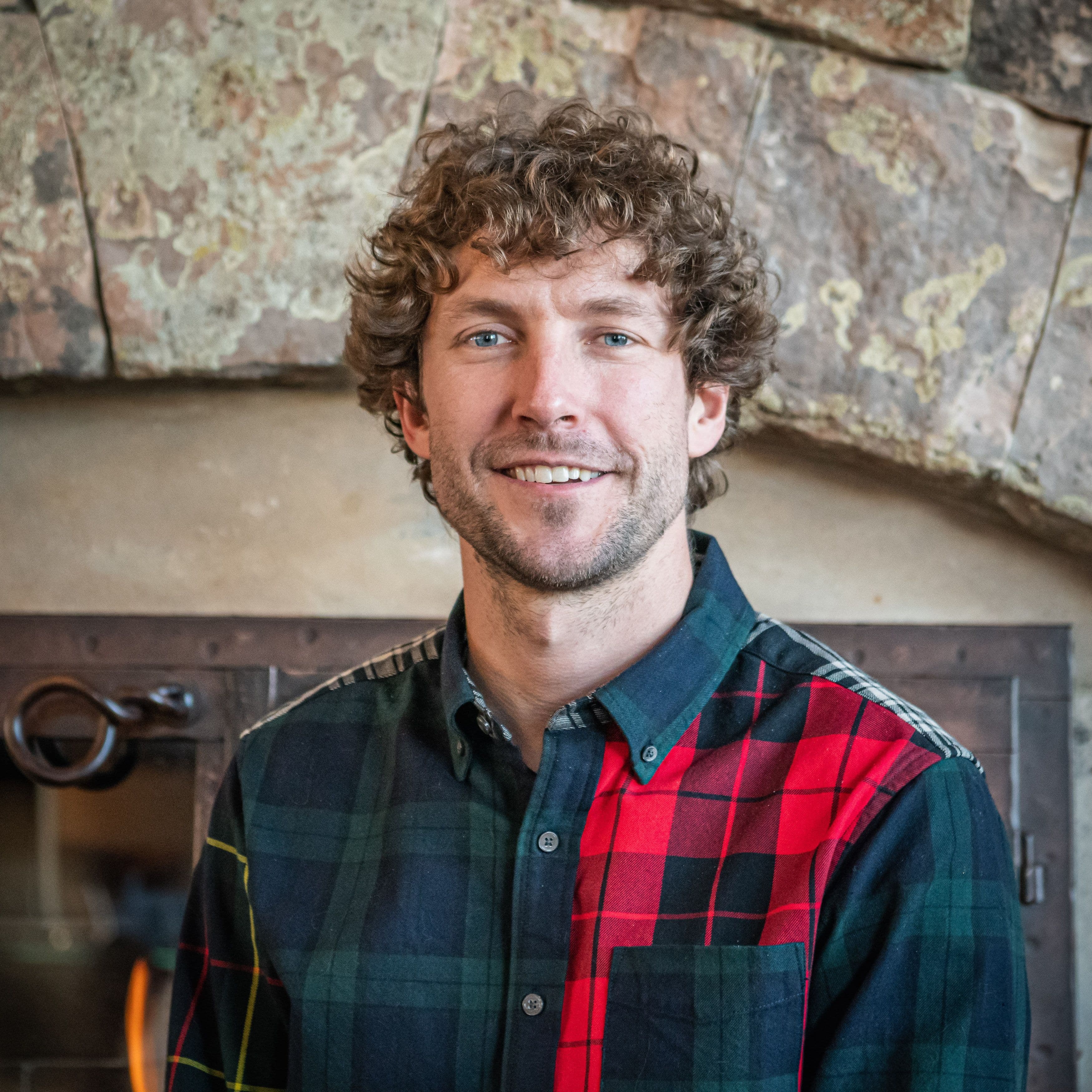
Lightweight Migrations
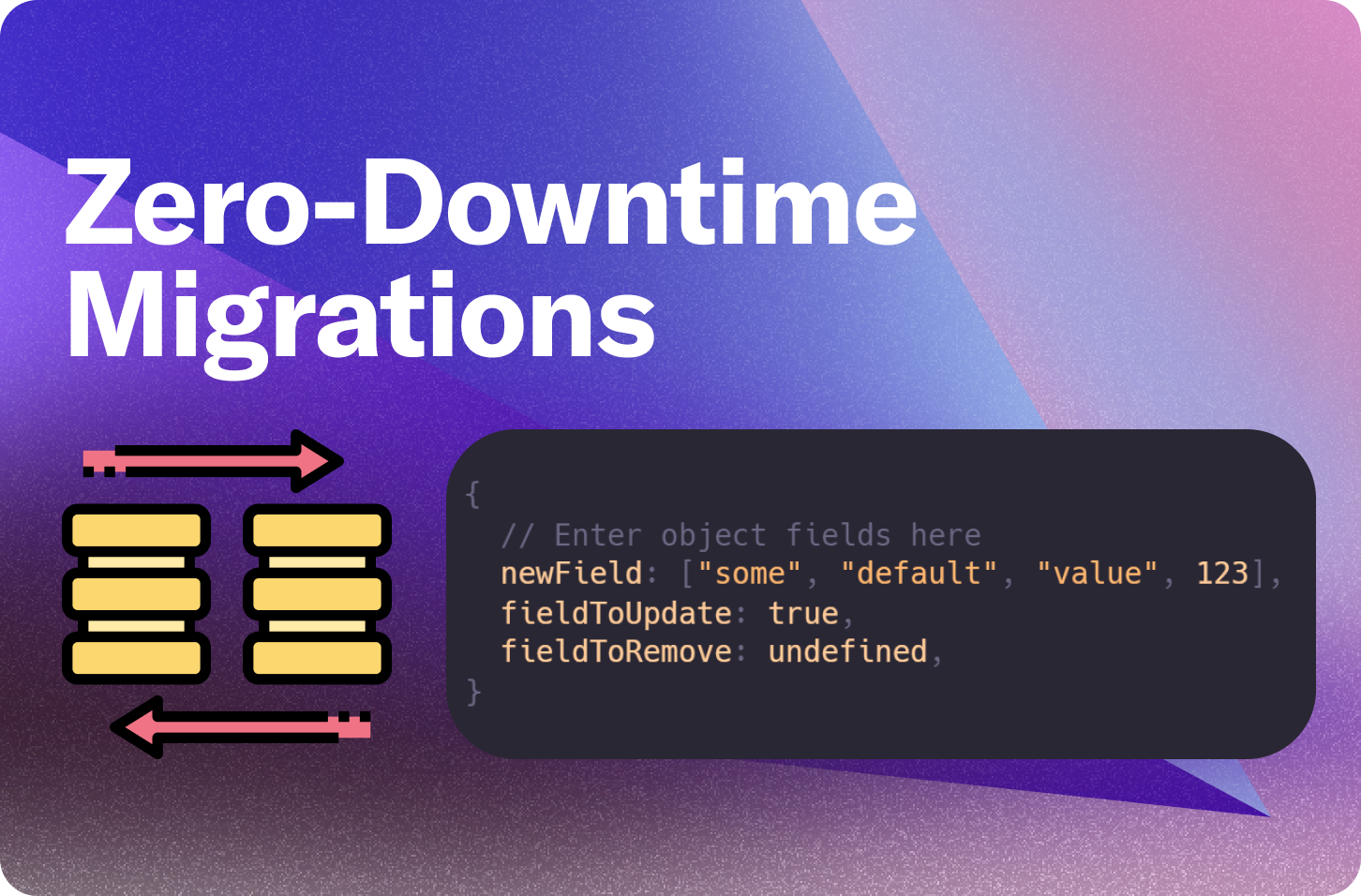
Want to make a small change to every document in your database? For instance, setting some new field to some value, without breaking your app for users for "scheduled maintenance"? You'll need to run a migration. In this post we'll look at how to apply lightweight changes to all of your documents without downtime, and without writing and deploying code to run lightweight migrations.
What are migrations?
Migrations are the process to change data in a database from one format to another. Read here for an overview of migrations.
Online migrations recap
If your app has a ton of data, it becomes infeasible to run this migration atomically in one transaction. In order to stay "online" while changing data, you need to write code that handles the old and new format of data temporarily. For example, to add a new field you can add an "optional" field to your schema, and update your code to handle it being set or unset. In this post we discussed how to use mutations to paginate over your data, applying some transformation.
This can be tedious if you're trying to quickly iterate on your development instance. Can we do this without deploying mutations?
Using bulk edit on the dashboard to patch documents
The Convex dashboard allows you to bulk edit documents.1 You can manually select certain documents, or you can click the checkbox above all documents to select all documents. When you bulk edit, you are given a JSON editor for what "patch" to apply to each document in the database. For example, you can apply this patch:
1{
2 // Enter object fields here
3 newField: ["some", "value", 123],
4 fieldToUpdate: true,
5 fieldToRemove: undefined
6}
7
Let's say there's these documents already in the database:
1{ fieldToUpdate: false, fieldToRemove: "foo", someField: 123 }
2{ fieldToUpdate: true, fieldToRemove: "bar", someField: 456 }
3
Applying this patch will update the documents to:
1{ fieldToUpdate: true, newField: ["some", "value", 123], someField: 123 }
2{ fieldToUpdate: true, newField: ["some", "value", 123], someField: 456 }
3
Note that someField
was not affected, since it was not specified in the patch.
Race conditions? Not a problem
Thanks to Convex's transactions, featuring serializable isolation, mean that if another mutation updates one of these documents at the same time as this update is being applied, one of the changes will be applied first, and the other mutation will be retried. This means you don't have to worry about some change being lost along the way.
You are still responsible for your code handling the case where newField
hasn't been set yet, or fieldToUpdate
is still false
. However, you'll never see a document where newField
is set and fieldToUpdate
hasn't been set to true
yet.
If your data can't all be updated in one transaction (if you have many thousands of documents), read this post for how to break up the change into a paginated mutation, and for best practices around dual-write vs. dual-read strategies while changing schemas. In these cases, you'll be responsible for handling the case where you fetch some documents that have been updated, and some that haven't yet been updated, so some documents might have the new field and some may not.
Invalid schema? Not a problem
If you try to apply the above patch but it doesn't match your defined schema (and you haven't turned schema validation off), the change will fail to apply to avoid writing invalid data. Here are some examples of schemas that would be invalid with the above patch:
newField
is missing
1// in convex/schema.ts
2export default defineSchema({
3 myTable: defineTable({
4 // newField needs to be specified to be set
5 fieldToUpdate: v.boolean(),
6 fieldToRemove: v.optional(v.string()),
7 someField: v.number(),
8 });
9});
10
newField
isn't optional
1// in convex/schema.ts
2export default defineSchema({
3 myTable: defineTable({
4 // newField needs to be optional when it's added to the schema
5 // since none of the documents have the value set yet.
6 newField: v.array(v.union(v.string(), v.number())),
7 fieldToUpdate: v.boolean(),
8 fieldToRemove: v.optional(v.string()),
9 someField: v.number(),
10 });
11});
12
newField
is the wrong type
1// in convex/schema.ts
2export default defineSchema({
3 myTable: defineTable({
4 // newField doesn't allow numbers in the array.
5 // The inserted value needs to match the schema definition.
6 newField: v.optional(v.array(v.string())),
7 fieldToUpdate: v.boolean(),
8 fieldToRemove: v.optional(v.string()),
9 someField: v.number(),
10 });
11});
12
fieldToRemove
isn't optional
1// in convex/schema.ts
2export default defineSchema({
3 myTable: defineTable({
4 newField: v.optional(v.array(v.union(v.string(), v.number()))),
5 fieldToUpdate: v.boolean(),
6 fieldToRemove: v.optional(v.string()),
7 someField: v.number(),
8 });
9});
10
Precautions
I would recommend using this functionality primarily on your "Dev" instance and not "Prod" for a few reasons:
- Accidental changes leading to data loss. If you accidentally set "email" to
""
on all of your documents in production, you have just lost all of your email user data. The dashboard will warn you before applying changes to production to help reduce the chances of accidentally changing production data, but it won't prevent you from making the changes if you bypass the warning. - Documenting changes. By committing the code to source control and deploying the code that you're running, you'll have a clear history of what changes you made to your data. Others can use this code to update other instances, such as any staging or other production-adjacent deployments.
- Testing changes. By using a migration helper you can print out intermediate status, and run a "dry run" first to test that you're making the expected changes (by throwing an exception in a mutation, the changes will be rolled back and won't apply).
Complicated migrations?
If you need to do more than set literal values - for instance if you're changing from having an pendingInvite: v.boolean()
field to status: v.union(v.literal("active"), v.literal("invited"))
, you'll want to write code. Check out migration helper about writing migration helpers like:
1export const updateActiveToStatus = migration({
2 table: "users",
3 migrateDoc: async (ctx, user) => {
4 await ctx.db.patch(user._id, {
5 status: user.pendingInvite ? "invited" : "active",
6 active: pendingInvite,
7 });
8 },
9});
10
Summary
You can patch all of your data in your database table with the bulk edit feature on the Convex dashboard, without writing migration code. If you're interested in more dashboard features, check out the docs or this post.
Footnotes
-
It is currently limited to making changes in a single transaction, so if you have 8,192 documents or more, you'll want to follow the advice of this post. We may allow the dashboard to patch all data in batches soon. ↩
Convex is the backend platform with everything you need to build your full-stack AI project. Cloud functions, a database, file storage, scheduling, workflow, vector search, and realtime updates fit together seamlessly.