
Testing Your App: How to Generate Fake Data
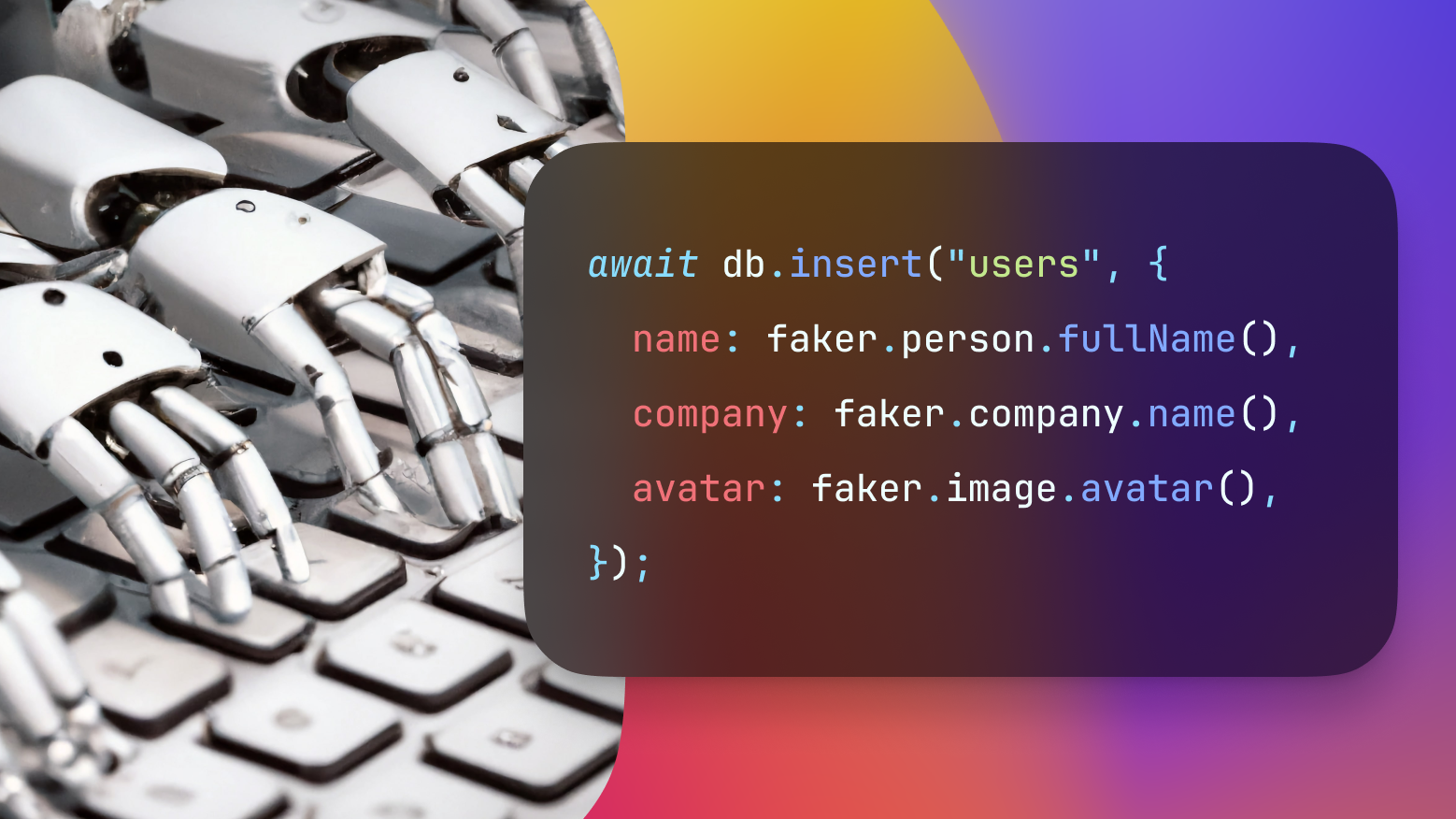
Are you setting up a new development environment and would like to fill it with sample data instead of it being empty? Or perhaps you want to make sure that your UI looks good when you have more than a few rows in your database?
You could create many rows by yourself, but it is tedious if you want good-looking and numerous results. In this article, we’re going to discover a much better way to seed your database with sample data.
Install Faker
We’re going to use a library called Faker. It provides helpful functions to generate realistic values in large quantities.
Before we begin, make sure you have a Convex project set up on your machine. If you don't have one already, you can create one using the Quickstart guide.
Then, install Faker using the following command:
npm install @faker-js/faker
Create an Internal Mutation
I will start by creating a new mutation in my Convex app by writing the following code in a file named convex/users.ts
:
import { internalMutation } from "./_generated/server";
export const createFake = internalMutation(async (ctx) => {
// …
});
Marking the mutation as internal ensures that users of my app can’t call it. I will still be able to call it myself from the dashboard or the Convex command-line tool.
Insert Fake Data
Now, we’re ready to start inserting data. You can consult the Faker documentation to know which data types it can generate:
The Faker documentation.
For my project, I need a list of users that each have a name, a company, and an avatar. I will use the corresponding types to create 200 new users:
import { faker } from '@faker-js/faker';
import { internalMutation } from "./_generated/server";
export const createFake = internalMutation(async (ctx) => {
// Initialize Faker with a random value
faker.seed();
for (let i = 0; i < 200; i++) {
await ctx.db.insert("users", {
name: faker.person.fullName(),
company: faker.company.name(),
avatar: faker.image.avatar(),
});
}
});
In the beginning of the function, we call faker.seed()
so that Faker generates different data each time we call our function. We need it because Faker initializes its random number generator statically. When using Convex, this will happen every time you push new code, not every time you call a function.
Now that we’ve written our function, we can run it using npx convex run
in the command line. You can also run it from the Convex dashboard instead.
npx convex run users:createFake
When opening my users
table in the Convex dashboard, I can see that I’ve successfully created 200 new users:
My fake users in the dashboard.
And when opening my app, I can see that not only do I see all my fake users, but also that they all have avatars!
The new users in my sample app.
Summary
We’ve seen how to create fake data using the Faker library and use it to create rows in the Convex database. To learn more advanced ways to generate fake data, you can read the Faker documentation.
Convex is the backend application platform with everything you need to build your project. Cloud functions, a database, file storage, scheduling, search, and realtime updates fit together seamlessly.