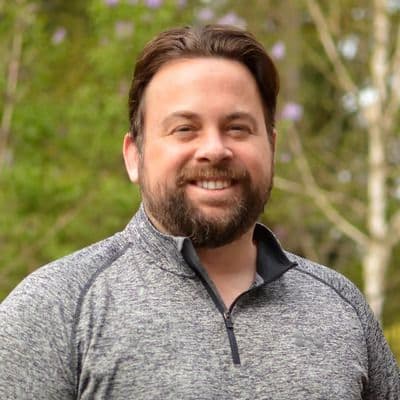
Edge to Butt: Wrappers as "Middleware"
Loading...
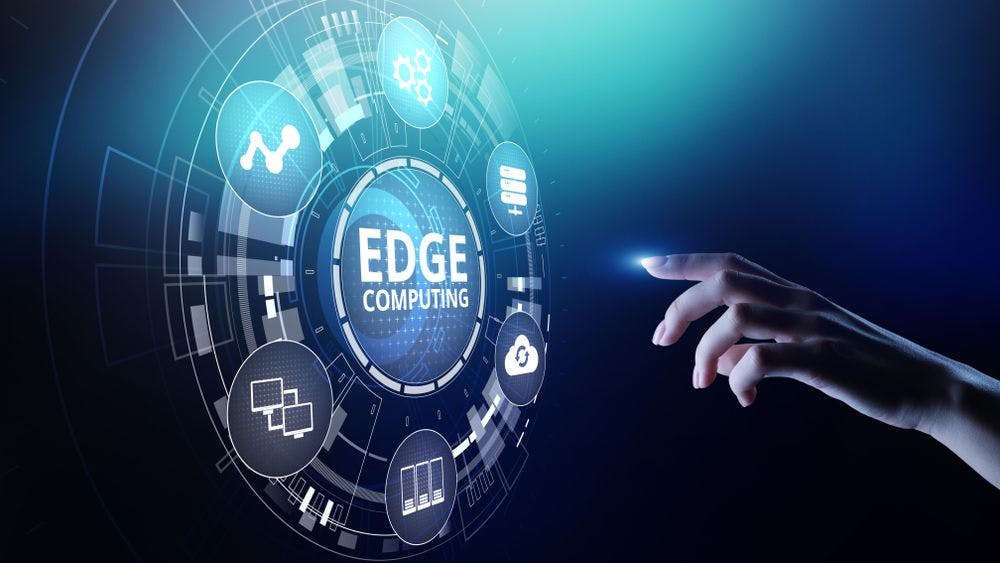
I loved reading the informative and useful Wrappers as Middleware: Authentication from Ian Macartney. But it’s time to get serious and Enterprise in here. Let’s focus on a piece of Convex middleware real projects need.
Years ago, an inspired soul created a “Cloud to Butt” movement wherein millions of Internet denizens installed a cheeky Chrome extension. Within Chrome, this extension transformed all web content uses of the word “cloud”, or “the cloud”, to "butt", “my butt”, and so on. Resulting in childishness like this:
Screenshot
This whole phenomenon was clearly a cheap attempt to capitalize on our collective cynical, weary leeriness at the growing ubiquity of the word “cloud”. Everything we used to just call the Internet, or servers, or whatever, started being labeled “the cloud” one day by breathless digital marketers. So we responded by channeling our inner seven-year-olds and using potty humor.
But it’s not 2014, it’s 2023. We’ve all matured, and we’ve even come to embrace the term cloud as a warm friend–of course the cloud is here, and it generously runs everything for us for an oh-so reasonable fee, and we embrace it! We wouldn’t dream of offending the cloud!
Now the new intruder we’re rolling our eyes at is “the edge”. The edge is nothing like the cloud. It’s mysterious and coming for us. Everyone is starting to say it and we’re starting to feel inadequate that we’re not using it enough or talking about it the right way at parties!
Problem solved.
Introducing the newest piece of Convex middleware, Edge-to-Butt. Just drop this little beauty into your Convex queries and mutations, and your website will thumb its nose at this snot-nosed newcomer they're calling “the edge”.
First, let’s write a function that traverses any object or array recursively and looks for strings:
1// Recursively explore objects/arrays and scalar values, looking for strings
2// to transform from 'edge' into 'butt'.
3function buttify(value: any): any {
4 const isArr = Array.isArray(value);
5 if (isArr) {
6 // recurse for all items in the array
7 value.forEach((v, i) => {
8 value[i] = buttify(v);
9 });
10 return value;
11 }
12 const valueType = typeof value;
13 if (valueType === "object") {
14 for (var key of Object.keys(value)) {
15 // recurse for all fields on the object
16 value[key] = buttify(value[key]);
17 }
18 return value;
19 }
20 if (valueType === "string") {
21 // String! replace "edge" with "butt", as one does.
22 return value.replace(/(^|\W)(edge)(\W|$)/gim, caseStableButtification);
23 }
24 return value;
25}
26
Now, the key to this whole system is this caseStableButtification
function. This is Enterprise software, so we need to get the details right. We want to make sure we only change the word ‘edge’, not ‘ledge’. And we want to preserve the case, so if someone says “Edge computing is the future”, we’ll want a capital B on that baby.
Here’s what that function looks like, operating on the groups of our matched regex:
1// Convert 'edge' to 'butt' while preserving case and surrounding syntax.
2function caseStableButtification(
3 _: string,
4 prefix: string,
5 edge: string,
6 suffix: string
7): string {
8 const fixEdge = (s: string): string => {
9 var buttLetters = [...s].map((l, i) => {
10 if (l.toLocaleUpperCase() === l) {
11 return BUTT.charAt(i).toLocaleUpperCase();
12 } else {
13 return BUTT.charAt(i);
14 }
15 });
16 return buttLetters.join("");
17 };
18 return prefix + fixEdge(edge) + suffix;
19}
20
Finally, we need our Convex wrapper, and we'll use it on the standard listMessages
query from the Convex tutorial:
1/**
2 * Wrapper for Convex query or mutation functions turns all use of "edge" to
3 * butt.
4 *
5 * @param - func: your Convex query function.
6 * @returns A return value with all strings having "edge" transformed
7 * into "butt".
8 */
9export const withEdgeToButt = (func: any) => {
10 return async (...args: any[]) => {
11 let result = await func(...args);
12 buttify(result);
13 return result;
14 };
15};
16
17// Retrieve all chat messages from the database with a sprinkle of 7-year old humor.
18export default query(
19 withEdgeToButt(async ({ db }: any) => {
20 console.log("getting messages again");
21 return await db.query("messages").collect();
22 })
23);
24
On the edge of your seat?
Are we ready for production? You tell me:
Screenshot of the final app
I’m sure for many of you, this was the final Convex capability you were waiting for before taking the leap and using Convex on your next project. Please be patient with the team while we manage the influx of interest. Your call is important to us.
Convex is the sync platform with everything you need to build your full-stack project. Cloud functions, a database, file storage, scheduling, search, and realtime updates fit together seamlessly.