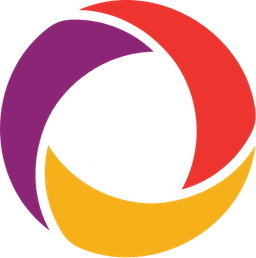
Event Driven Programming: A Definitive Guide
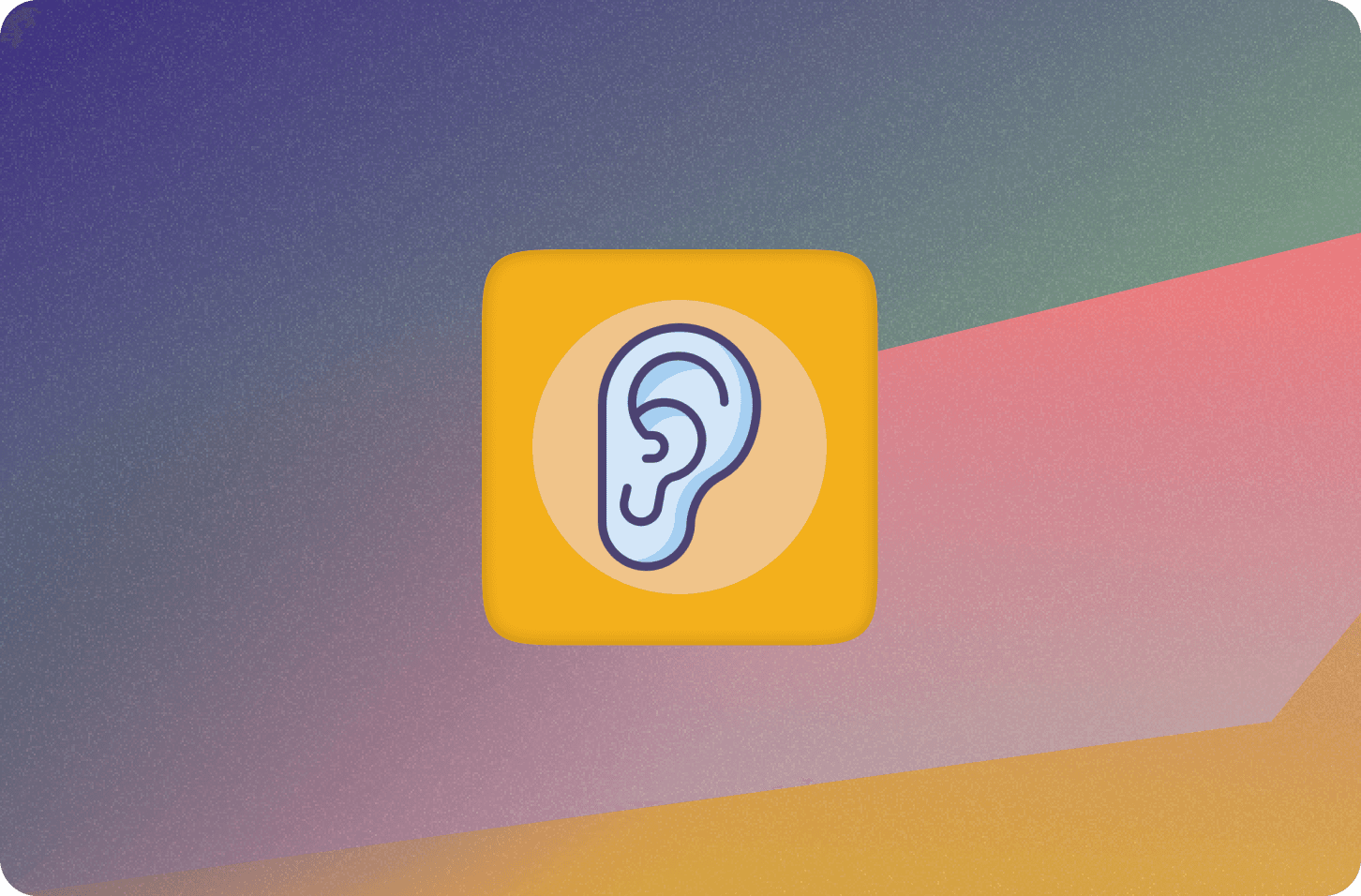
Event-driven programming. Yeah, you’ve heard of it, but how does it fit into your development process? Or should it? Event-driven programming (EDP) focuses on events—specific actions an application listens for and responds to— to enable you to create highly responsive, scalable applications.
Event-driven architecture (EDA), a key concept in modern software development, is especially useful for developing distributed systems, microservices, and applications that must react quickly and process data streams efficiently.
If you’re struggling to make your apps more responsive or scalable, an event-based programming model might be your answer. Read on as we explore the basics of EDP and how it can improve your development process.
What is Event-Driven Programming?
Event-driven programming enables decoupled components to communicate by producing, detecting, consuming, and reacting to events. An event-driven program’s flow is determined by events such as user actions, system changes, sensor outputs, or messages from other programs. Instead of following a linear sequence of instructions, the program waits for and responds to these events.
Events interact with three other key components of an event-driven system: event sources, event listeners, and event handlers.
- Event sources (producers) generate and publish events such as button clicks or sensor data. They notify the application about these events without knowing which components are listening for or handling the events.
- Event listeners (or subscribers) register for specific events and respond when they occur. When an event occurs, the listener is notified and calls the corresponding event handler, which executes the code to handle the event.
- Event handlers, the functions or methods processing the event, perform tasks such as updating the user interface, making API calls, or triggering other events.
So let’s break this down with a practical TypeScript example.
How Event-Driven Programming Works
Consider a simple scenario: a user interface where a button click triggers an action. The button is the event source, the event listener is registered to detect the click event, and the event handler performs an action.
- Event Source: The button that the user clicks that generates the event.
1// Event Source: The button element
2const button = document.getElementById('fetchButton') as HTMLButtonElement;```
3
- Event Handler: this is the callback function that is executed in response to an event. In this example, it fetches data from an API and updates the UI.
1// Event Handler: Function to fetch data and update the UI
2const fetchData = async () => {
3 try {
4 const response = await fetch('https://api.example.com/data');
5 const data = await response.json();
6 displayData(data);
7 } catch (error) {
8 console.error('Error fetching data:', error);
9 }
10};
11
12// Function to update the UI with fetched data
13const displayData = (data: any) => {
14 const displayElement = document.getElementById('dataDisplay') as HTMLDivElement;
15 displayElement.textContent = JSON.stringify(data, null, 2);
16};
17
- Event Listener: listens for the button click event and specifies which event handler to call.
1// Event Listener: Setting up the listener for the button click event
2button.addEventListener('click', fetchData);
3
- **Event Queue: **The listener places the event in a queue where they are stored until they can be processed. The queue also helps to manage concurrency and synchronization issues that arise.
- Event Loop: runs in the background, constantly checking the queue for events and calling the corresponding event handler.
In summary, event-driven programming is all about using event sources to generate events, listeners detect them, and handlers to process the events.
Now, let's look at the benefits of using event-driven architecture.
Benefits of Using Event-Driven Architecture
Event-driven architecture (EDA) brings a ton of advantages, making it a go-to choice for building modern, responsive, and scalable apps. Here are some key benefits:
Improved application responsiveness
Event-driven systems react to events as they happen. This means no more polling for changes, no waiting for a specific sequence of actions, and minimal lag in data synchronization. Events are processed asynchronously which means you can handle them in real-time.
Enhanced scalability and flexibility
In a traditional monolithic setup, tightly coupled components make scaling a nightmare. But with event-driven architecture, event producers and event consumers work independently. This lets you adapt and grow your system. Need to handle more events? Just add more event consumers. Your system can grow without you breaking a sweat.
Loose coupling
Loose coupling is a game-changer for modularity and maintainability. In event-oriented programming, event producers and consumers don’t depend on each other directly. Producers generate and publish events without worrying about who’s consuming them, while consumers subscribe to events without caring who produced them. Because of this separation, changes in one part of the system don’t affect the whole app. You can update or replace individual components without a massive overhaul.
For those new to event-driven architecture, there can be some confusion about the differences between event-driven and reactive programming. We’ll look at that next.
Event-Driven vs. Reactive Programming
Event-driven and reactive programming both improve system responsiveness and efficiency but have different philosophies and approaches. Let’s break down the differences so you can pick the right one for your project.
Design philosophy and approach
While event-driven programming decouples components so each part can operate independently and respond to events as they happen, reactive programming focuses on data flow and automatic propagation of changes. In EDP, events signal changes, listeners subscribe to specific events, and handlers process them.
Reactive programming, on the other hand, creates data streams representing ongoing sequences of events. Components subscribe to these streams and react to data changes, making interactions more fluid.
Data vs. Process Orientation
Event-oriented programming is all about processes triggered by events, with state changes handled by event handlers. It’s ideal for scenarios where specific actions are needed based on user interactions or system events, like updating inventory when a new order is placed.
Reactive programming treats data as a stream with components reacting to changes. It’s perfect for apps dependent on real-time data updates, like a stock ticker that shows price changes across all connected clients in real time.
Declarative vs. Imperative
By nature, event-driven programming is imperative, requiring detailed steps to process events and update the system state for more fine-grained control. The focus is on control flow and the sequence of events that drive an application's behavior.
The code in the example below defines how to handle a “click” event, fetch data, and update the user display. Each step is laid out clearly for direct control over the process.
Code Example:
1const button = document.getElementById('fetchButton');
2const displayElement = document.getElementById('dataDisplay');
3
4button.addEventListener('click', async () => {
5 const response = await fetch('https://api.example.com/data');
6 const data = await response.json();
7 displayElement.textContent = JSON.stringify(data, null, 2);
8});
9
Reactive programming is more declarative, focusing instead on what the data flow should look like, not the steps needed to execute it. Developers define relationships between data streams, and the system propagates changes automatically. This simplifies the code and increases readability, making complex data interactions more intuitive and easier to manage.
The code below defines the data flow: when a user clicks the button, the application fetches data and updates the display. The **switchMap **and **map **operators abstract the details, making the code more declarative and easier to follow.
Code Example:
1import { fromEvent } from 'rxjs';
2import { switchMap, map } from 'rxjs/operators';
3
4const button = document.getElementById('fetchButton') as HTMLButtonElement | null;
5const displayElement = document.getElementById('dataDisplay') as HTMLElement | null;
6
7if (button && displayElement) {
8 fromEvent(button, 'click')
9 .pipe(
10 switchMap(() => fetch('https://api.example.com/data').then(response => response.json())),
11 map(data => JSON.stringify(data, null, 2))
12 )
13 .subscribe(data => {
14 displayElement.textContent = data;
15 });
16}
17
Event handling
Event-driven code uses callbacks, promises, and event emitters to handle events and async operations explicitly and ensure predictable responses. Events can be processed synchronously or asynchronously.
Reactive programming uses the observer pattern and observables for handling async data streams. Compared to callbacks and promises, observables are a more powerful, flexible way to manage async data flows, supporting operations like filtering, mapping, and combining streams. This approach provides a higher level of abstraction and flexibility when handling events.
So in summary, use event-driven programming when you need explicit control of events and reactive programming when a seamless data flow is what you’re looking for.
Types of Event-Driven Architecture
Event-driven architecture comes in different flavors, each suited to various applications and use cases. Here are some common types, along with real-world examples:
Complex Event Processing (CEP)
Complex Event Processing (CEP) analyzes multiple events to identify patterns, combinations, and event correlations. CEP systems handle high volumes of events in real time, mine them for meaningful insights, and trigger actions based on complex event patterns. A financial trading app, for example, detects price changes in multiple event streams and automatically executes trades based on set rules.
Message Queue Architecture
In this setup, message queues manage communication between system components. Producers publish messages to a queue, and consumers process them asynchronously. A common example is an e-commerce system that queues orders while a separate service fulfills them. This balances the workload and lets the website front-end scale independently.
Pub/Sub (Publish/Subscribe) Messaging Services
In the Pub/Sub model, producers send events to a message broker, which distributes them to subscribers for async processing. Imagine an e-commerce platform using Google Cloud Pub/Sub: an "order created" event triggers microservices for inventory, billing, and notifications to handle stock updates, payments, and confirmations. This supports one-to-many event distribution where multiple consumers receive the same events.
Event Sourcing
The Event Sourcing pattern captures state changes as a sequence of immutable events, instead of storing the current state directly. This way, the system records every change and can reconstruct the current state by replaying events. This is a common approach in banking apps that record each transaction as an event to compute the current balance.
Event Stores
These specialized databases, built for storing and retrieving event data, are the backbone of event-sourcing architectures. Event stores are often used in financial applications where audit trails are important for transaction history, fraud detection, and detailed account statements.
Understanding the strengths and use cases of each model can help you choose the right architecture for your event-driven program.
5 Common Use Cases for Event-Driven Programming
With event-based programming, you can create modern software systems for all sorts of scenarios. Here are some common use cases:
- Logging and monitoring systems: Monitoring and analytics services subscribe to events from producers like microservices, then process logs, trigger alerts, and generate reports based on predefined rules.
- Real-Time notification services: Notification services listen for events from a project management tool, for example, to send push notifications, emails, or SMS messages to the recipients in real-time.
- Network request handling: An API gateway queues incoming client requests and processes them asynchronously during high-traffic periods without affecting application responsiveness.
- Microservice-based Apps: Microservices-based e-commerce apps publish order creation events while other microservices subscribe to handle order confirmations, inventory updates, and notifications.
- User Interface Interactions: Event-oriented programming is the backbone of modern GUIs. User actions like button clicks, mouse movements, or keyboard inputs trigger events handled by the app’s event loop. Event handlers respond to update the UI dynamically without reloading the page.
Many of these use cases apply to both small and large-scale applications, but the benefits can vary based on your project's scale and complexity. Let’s look at the benefits and challenges of event-based programming in these scenarios.
Suitability for Small vs Large Applications
For small applications, event-driven programming can streamline processes and improve responsiveness without heavy overhead. Decoupled components make the app more modular and easier to maintain. Even in small projects, using events for user actions, data changes, and network requests keeps the codebase clean and manageable.
Large-scale applications are where the event-driven model shines. Its modular nature keeps things tidy, making it easier to manage the complexity of tons of events at scale. Decoupling components also simplifies communication and makes it easier to add, remove, or tweak features. EDP’s async nature is also perfect for handling user interactions and real-time data streams, allowing large apps to scale horizontally.
Considerations for Both Scales: For very small apps, the overhead of setting up event handlers might not be worth it; a linear approach could work better. In large-scale applications, ensuring effective communication and proper event handling requires careful planning and solid infrastructure. Developers must consider event ordering, error handling, and performance optimization to keep the system stable and efficient at scale.
Introduction to Event Libraries
Event-based programming thrives on libraries and frameworks that handle event management, state management, and asynchronous processing. Here’s a look at some of the most popular ones:
- RxJS (Reactive Extensions for JavaScript): a powerhouse for composing async, event-based programs using observable sequences. It handles complex async data streams effortlessly, making it perfect for reactive UIs, real-time data, and intricate workflows in JavaScript and TypeScript.
- EventEmitter (Node.js): a core Node.js module that offers a lightweight, event-driven architecture for emitting and handling events. It’s perfect for async communication, handling I/O operations, building modular code, and creating event-driven architectures in Node.js apps.
- Apache Kafka: A distributed event streaming platform designed for high-throughput, low-latency data feeds. It uses the publish-subscribe model to manage real-time data streams. Kafka’s architecture supports several use cases, including streaming analytics, high-performance data pipelines, and mission-critical applications.
- Redux (React): a state management library that uses a unidirectional data flow to ensure traceable and consistent state transitions. Its core principles are a single source of truth (the store), a read-only state, and pure functions. With middleware like Redux Thunk to handle async actions, Redux is a go-to for managing complex state in large React apps.
State management is key in event-driven architectures to ensure consistent state changes across complex apps. Libraries reduce bugs, improve readability, and simplify management. Tools like Redux DevTools help track changes, making optimization and troubleshooting easier.
Managing Asynchronous Events
Debugging asynchronous events in event-driven architectures can be tricky because of the decoupled nature of components and unpredictable order of event processing. Logging and monitoring tools capture detailed logs that provide insights into the system’s behavior. This helps developers ensure correct event processing which leads to faster issue resolution.
Key considerations for debugging asynchronous events include:
- Implement comprehensive logging at various levels (application, framework, infrastructure) to capture relevant events, state changes, and errors.
- Use structured logging formats like JSON for easy parsing and querying.
- Integrate with centralized logging and monitoring platforms that offer search, analysis, and visualization capabilities.
- Correlate events across distributed components using unique identifiers or tracing mechanisms.
- Use monitoring tools to track key performance metrics, detect anomalies, and generate alerts.
- Employ debugging frameworks or libraries with tools to inspect event streams, replay events, and visualize event flows.
Automatic state synchronization services make debugging easier as they ensure all parts of the application have a consistent view of its data. Convex’s open-source backend offers real-time database synchronization that ensures all clients see the same data simultaneously. This is essential for apps that require immediate updates and consistent state management across multiple users and devices. Other popular state synchronization options include Akka Cluster (Scala/Java), Hazelcast (Java), and Redis (Multi-language).
Next, let’s tackle some common challenges in EDP, then wrap up with some final thoughts on event-driven programming.
Addressing Common Challenges in Event-Driven Programming
Event-based programming is awesome, but it comes with its own set of hurdles. Here are some practical strategies to help you tackle these challenges:
Implement event sourcing to derive state from event sequences: Event sourcing is like your app’s time machine. It records state change as a separate event to give you a complete, unchangeable history of state transitions. This log ensures data consistency, compliance, and easier troubleshooting. Developers can replay events to reconstruct past states or fix issues.
Use CQRS to separate read and write operations: Command Query Responsibility Segregation (CQRS) splits read and write operations into distinct models. This helps boost scalability, performance, and data consistency, especially in large apps. By separating models, you can optimize and scale each independently for its specific workload.
Create utility functions or classes for repetitive event handling logic: Create reusable utility functions or classes to handle similar events across multiple components. This keeps your codebase clean, reduces redundancy, and makes maintenance a breeze.
Use frameworks for built-in support: Frameworks like Spring for Java and Django Channels for Python support event-driven architectures. They handle async communication, event lifecycles, and data consistency across distributed components, letting you focus on building awesome features while they manage the heavy lifting.
Apply design patterns like the Observer pattern for loose coupling: The Observer pattern keeps things loosely coupled. It lets objects (subjects) notify dependents (observers) of state changes without creating dependencies, making inter-component communication simpler.
Use BaaS platforms to reduce boilerplate code and enhance event handling: Backend-as-a-Service (BaaS) platforms like Firebase, AWS Amplify, and Convex simplify event-based programming by handling state synchronization, event distribution, and load balancing. Convex’s open-source backend offers real-time data reactivity and streamlined async workflow management that reduces boilerplate code and configuration.
Final Thoughts
Event-driven programming is a game-changer for creating and maintaining responsive applications. Whether it's managing asynchronous events, applying design patterns like the Observer pattern, or using BaaS platforms, EDP addresses the modern software challenges developers face.
Convex takes this a step further with real-time data reactivity, simplified asynchronous workflow management, and built-in functionalities that reduce boilerplate code and let your team focus on delivering value to your customers.
Learn more about how Convex can streamline your event-driven processes here.
Convex is the sync platform with everything you need to build your full-stack project. Cloud functions, a database, file storage, scheduling, search, and realtime updates fit together seamlessly.