
Introducing Convex for Android
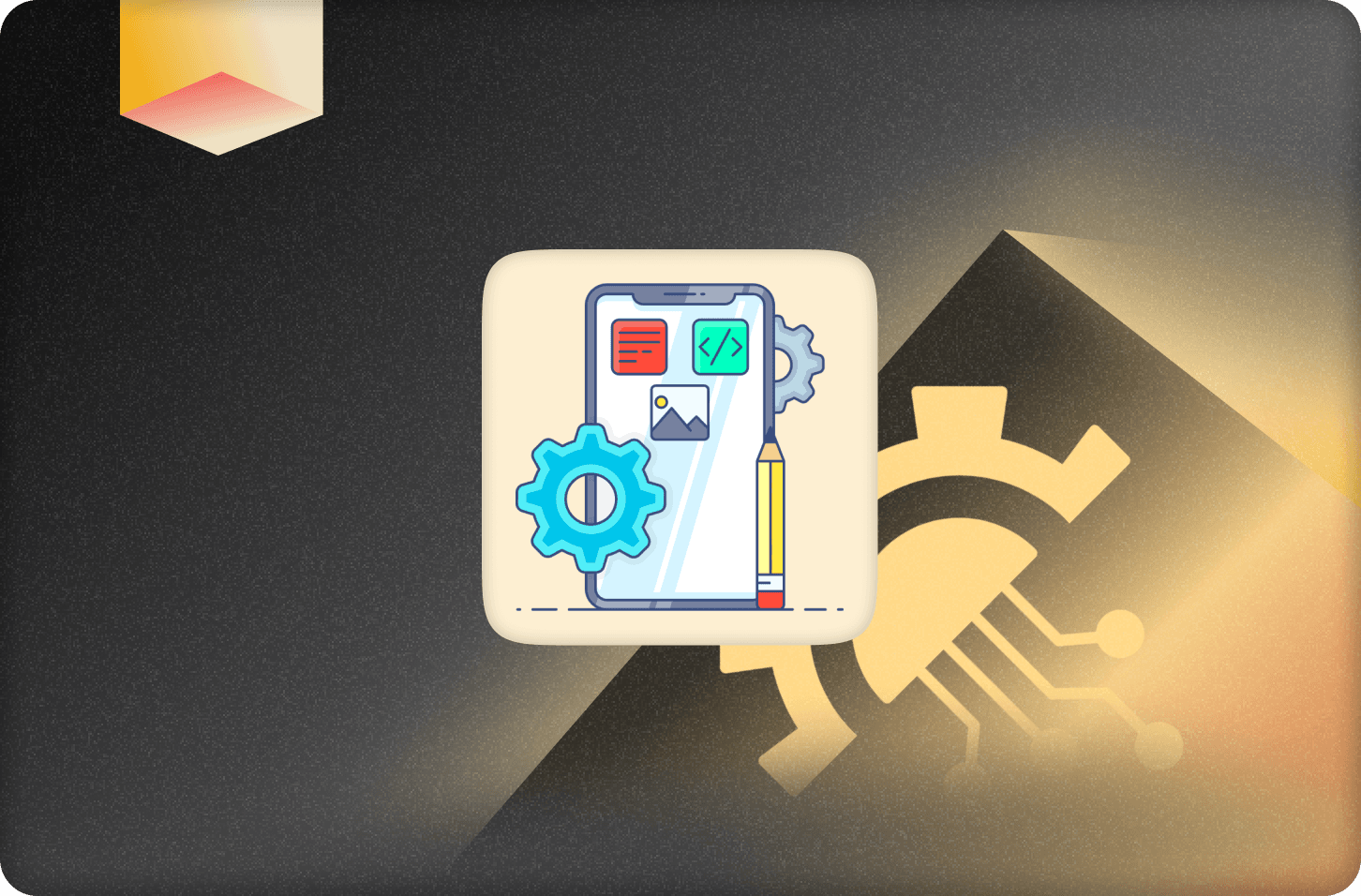
On behalf of the team at Convex, I’m happy to announce the initial release of Convex for Android!
Over the last 10 years, I’ve had the chance to help build some of the biggest Android apps in the world. In a lot of ways, I grew as a developer along with the Android platform as it has matured. Like any growing process, there were bumps along the way, but by and large I have learned a lot about what good application and API design looks like.
Recently I’ve had the exciting opportunity to work with the team here at Convex to bring mobile support to their reactive backend platform. It was my goal to create an API that both feels natural to Android developers who have kept up with modern application architecture principles, as well something that feels like a natural fit in the Convex ecosystem. Ideally it should disappear into the background as your app takes shape around it.
What follows is a walkthrough of how you can integrate Convex for Android into an app written in Kotlin.
Getting started
It’s really easy to hack together a quick example app with the library, as we demonstrate in the Android quickstart. If you want to go deeper and see how to structure a more realistic application with Android and Convex, read on to learn how a chat app like the one in the official Convex tour might be built. You’ll learn the fundamentals to create something that works like this:
ConvexClient - your data source
At the heart of Convex for Android is the ConvexClient
. It wraps the official convex-rs Rust client and handles the connection and communication with the backend. Your application should keep a single reference to a ConvexClient
and use it whenever it needs to communicate with the backend. To create a client, simply pass it the Convex deployment URL you want it to connect to.
1val convex = ConvexClient(DEPLOYMENT_URL)
2
ConvexClient
provides methods like mutation
to trigger updates to your data based on passed in arguments and subscribe
to reactively receive results from a Convex query.
Kotlin data classes for your data
It’s idiomatic to represent structured data as a data class
in Kotlin. Not surprisingly they’re a great fit for the chat messages our app will send and receive and they work well with the ConvexClient
too. Here’s a short data class
for the chat messages:
1@Serializable
2data class ChatMessage(
3 val author: String,
4 val body: String,
5)
6
The class is @Serializable
so it can be marshaled across the FFI boundary between the Kotlin and Rust layers of the library. The author
and body
properties allow the ChatMessage
to match the “shape” of the data stored in the backend messages
table.
A Repository to wrap ConvexClient
Now that we have our data source and a class to represent the data, we need somewhere to tie them together. In a modern Android application that is done in a Repository class. Our ChatRepository
will contain the business logic required to implement a simple chat client: sending and receiving messages.
1class ChatRepository(private val client: ConvexClient) {
2 /** Sends the given message to the backend. */
3 suspend fun sendMessage(message: ChatMessage) {
4 client.mutation(
5 "messages:send",
6 mapOf(
7 "body" to message.body,
8 "author" to message.author,
9 )
10 )
11 }
12
13 /**
14 * Subscribes to a Flow of messages from the backend.
15 *
16 * The Flow will be updated whenever a new message is posted.
17 */
18 suspend fun getMessages(): Flow<Result<List<ChatMessage>>> =
19 client.subscribe<List<ChatMessage>>("messages:list")
20}
21
A typical Repository layer will likely be pretty thin when using Convex. The ConvexClient
provides fairly rich and high level functionality that the Repository exposes with a slightly more friendly API. If you want, you can extract an interface for your Repository and use it in place of concrete implementations. That makes it easier to switch to different data storage implementations (like a fake for testing) while keeping your UI layer blissfully ignorant of those lower level details.
That’s actually about it for the Convex-specific integration! The rest of the walkthrough shows how to neatly wire this functionality into a reactive Android UI.
A custom Application class for holding the Repository
It’s convenient to provide access to instances of your Repository class(es) in a custom Application class. The custom class can be accessed at runtime and used to pass the Repository instance to other code that needs it.
1class ChatApplication : Application() {
2 lateinit var repository: ChatRepository
3 override fun onCreate() {
4 super.onCreate()
5 val convex = ConvexClient(DEPLOYMENT_URL)
6 repository = ChatRepository(convex)
7 }
8}
9
A ViewModel to bridge the Repository and your UI
Whether you build your UI with standard Android Views or with a modern reactive style using Jetpack Compose, the core of your UI state should live in a ViewModel. The ViewModel is the source of truth for your UI. It provides all of the data to render it and methods to perform any functionality it exposes to users. The ViewModel will also contain the in-memory state for your UI, comprised of:
- Data received from the Repository for use in the UI
- Data received from the UI for eventual transmission to the Repository
To start, let’s create a ChatViewModel
to expose a custom UiState
object that will be consumed by the UI to update the appearance of the app with messages received from the backend and an outgoing message.
1data class UiState(
2 val outgoingMessage: String,
3 val messages: List<ChatMessage>,
4)
5
6class ChatViewModel(private val chatRepository: ChatRepository) : ViewModel() {
7 private val _outgoingMessage: MutableStateFlow<String> = MutableStateFlow("")
8 private val _uiState: MutableStateFlow<UiState> = MutableStateFlow(
9 UiState(
10 outgoingMessage = "",
11 messages = emptyList(),
12 )
13 )
14 val uiState: StateFlow<UiState> = _uiState
15}
16
The actual UiState
that is produced by the ViewModel will change over time; as new messages are received from the backend and as the user enters new messages to send.
Modeling changes to the data over time is a great fit for Kotlin’s Flow
type. If you’re not familiar with Flow
, think of it as a Future
or Promise
that can “complete” multiple times whenever the upstream producer of data emits a new value.
Above we use MutableStateFlow<String>
and MutableStateFlow<UiState>
which represent flows that will emit a given value
and always return their latest state to a consumer. In both uses, an initial value is passed in to be used as the value emitted by the flow before any real data is produced.
Let’s update the code to populate the uiState
flow with messages received from Convex.
1class ChatViewModel(private val chatRepository: ChatRepository) : ViewModel() {
2 init {
3 // When the ViewModel is initialized, collect the Flow of messages from
4 // the Repository and set a new _uiState.value each time the data changes.
5 viewModelScope.launch {
6 chatRepository.getMessages().collect {
7 incoming ->
8 _uiState.value = UiState(
9 messages = incoming.orElse(emptyList()),
10 outgoingMessage = ""
11 )
12 }
13 }
14 }
15}
16
That code is enough to ensure that the public uiState
value will always contain the latest messages from the backend. We can make use of that in a Jetpack Compose UI. The following code will observe changes to uiState
and automatically re-render the list of ChatMessage
values when there’s a change:
1@Composable
2fun MessageList(val viewModel: ChatViewModel) {
3 val uiState by viewModel.uiState.collectAsState()
4 LazyColumn {
5 items(uiState.messages) { message ->
6 Text(
7 text = message.body
8 )
9 }
10 }
11}
12
Receiving messages and rebuilding the UI is great, but let’s add the ability to send messages too. We already have a MutableStateFlow
in our ViewModel to store an outgoing message. Now we’ll add methods to update that value and send it to the backend.
1class ChatViewModel(private val chatRepository: ChatRepository) : ViewModel() {
2 /** Called as the user enters text to compose an outgoing message. */
3 fun updateOutgoingMessage(message: String) {
4 _outgoingMessage.value = message
5 }
6
7 /** Called to send a composed outgoing message. */
8 fun sendOutgoingMessage() {
9 viewModelScope.launch {
10 chatRepository.sendMessage(
11 ChatMessage(
12 author = "Android Demo User",
13 body = _outgoingMessage.value
14 )
15 )
16 // Clear the outgoing message after it's sent.
17 _outgoingMessage.value = ""
18 }
19 }
20}
21
Let’s hook those methods up in the UI. We’ll create a simple Row
with a TextField
and an ElevatedButton
.
1@Composable
2fun OutgoingMessage(val viewModel: ChatViewModel) {
3 val uiState by viewModel.uiState.collectAsState()
4 Row{
5 TextField(
6 value = uiState.outgoingMessage,
7 onValueChange = viewModel::updateOutgoingMessage
8 )
9 ElevatedButton(
10 onClick = viewModel::sendOutgoingMessage
11 ) {
12 Text(text = "Send")
13 }
14 }
15}
16
Now the OutgoingMessage
UI will send changes in the TextField
to the ChatViewModel
and will also update its state when new UiState
is emitted (e.g. outgoingMessage
cleared after send).
There’s a problem though. The UiState
exposed by the ChatViewModel
only updates when new messages are received from the backend.
The flow without combine
The TextInput
in the UI won’t reflect what’s typed on the keyboard. Let’s change it so it updates whenever the outgoingMessage
changes too.
Again, we’ll make use of Flow
and use the combine
operator to take the latest ChatMessage
values from Convex and input from the UI and create one flow of UiState
from their data.
1class ChatViewModel(private val chatRepository: ChatRepository) : ViewModel() {
2 init {
3 // When the ViewModel is initialized, combine the messages from
4 // the Repository and the the outgoing message into a new Flow and
5 // collect to build a new _uiState.value each time either source changes.
6 viewModelScope.launch {
7 combine(_outgoingMessage,
8 chatRepository.getMessages()) { outgoing, incoming ->
9 UiState(
10 messages = incoming.orElse(emptyList()),
11 outgoingMessage = outgoing
12 )
13 }.collect { uiState ->
14 _uiState.value = uiState
15 }
16 }
17 }
18}
19
There we go! Now when either _outgoingMessage
or chatRespository.getMessages()
receives new data, the UI will rebuild with the latest data from each source.
The flow with combine
Where to next?
The walkthrough above left out some details for the sake of brevity. The app in the demo video shown near the beginning is nicely styled and has support for signing in and signing out, and the walkthrough code left those details out.
If you’re interested in building out a compelling UI using Jetpack Compose, take a look at the official Jetpack Compose Basics codelab.
If you want more details on how to use Convex with Android, check out the official docs and the source code for a multi-screen example app with auth support called Workout Tracker.
As for me, I’m excited to keep working on bring Convex to more mobile platforms! Keep an eye out for a follow on release for iOS and let us know if you hit any bugs or have a feature request by filing an issue in the convex-mobile Github repo.
Convex is the backend platform with everything you need to build your full-stack AI project. Cloud functions, a database, file storage, scheduling, workflow, vector search, and realtime updates fit together seamlessly.