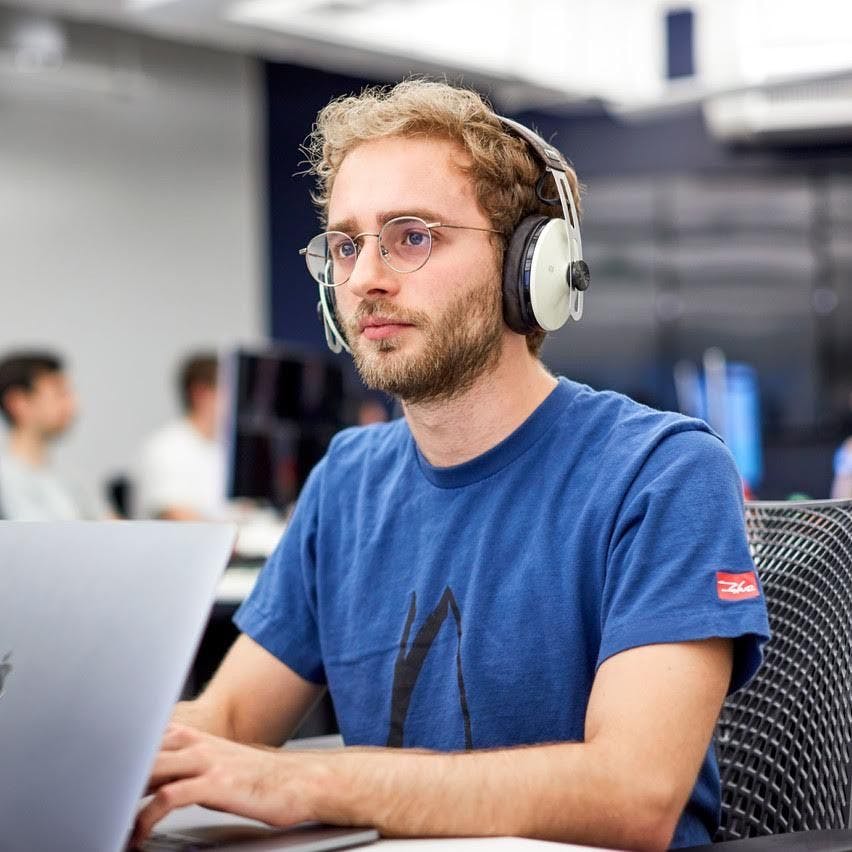
Testing React Components with Convex
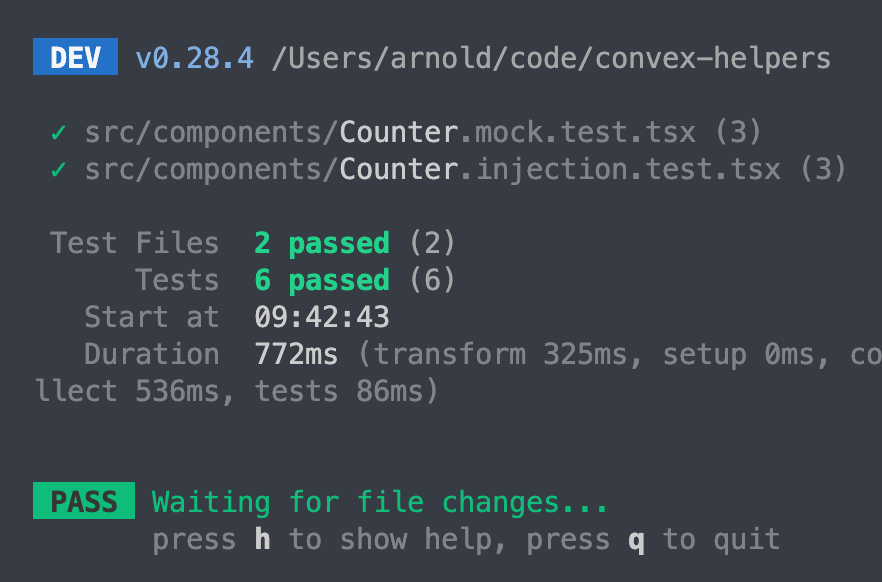
Oftentimes during testing we want to mock out our backend so we can unit test our UI components without talking to our actual server code.
In this article, we’ll explore options for testing React components that call Convex React hooks using mocking and dependency injection. To do this, I’ve written a sample TypeScript React app using the Vitest testing framework. The patterns presented in this post are also applicable to JavaScript apps and other frameworks.
Here, we have a React component that renders a counter value stored in Convex, and provides a button for incrementing the counter value by one.
1// See the full file here:
2// https://github.com/get-convex/convex-helpers/blob/main/src/components/Counter.tsx
3
4const Counter = () => {
5 const counter =
6 useQuery(api.counter.getCounter, { counterName: "clicks" }) ?? 0;
7 const incrementByOne = useCallback(
8 () => increment({ counterName: "clicks", increment: 1 }),
9 [increment]
10 );
11
12 return (
13 <div>
14 <p>
15 {"Here's the counter:"} {counter}
16 </p>
17 <button onClick={incrementByOne}>Add One!</button>
18 </div>
19 );
20};
21
22export default Counter;
23
Convex function details...
1// See the full file here:
2// https://github.com/get-convex/convex-helpers/blob/main/convex/counter.ts
3
4import { query } from "./_generated/server";
5import { mutation } from "./_generated/server";
6
7const getCounter = query(
8 async ({ db }, { counterName }: { counterName: string }): Promise<number> => {
9 const counterDoc = await db
10 .query("counter_table")
11 .filter((q) => q.eq(q.field("name"), counterName))
12 .first();
13 return counterDoc === null ? 0 : counterDoc.counter;
14 }
15);
16
17const incrementCounter = mutation(
18 async (
19 { db },
20 { counterName, increment }: { counterName: string; increment: number }
21 ) => {
22 const counterDoc = await db
23 .query("counter_table")
24 .filter((q) => q.eq(q.field("name"), counterName))
25 .first();
26 if (counterDoc === null) {
27 await db.insert("counter_table", {
28 name: counterName,
29 counter: increment,
30 });
31 } else {
32 counterDoc.counter += increment;
33 await db.replace(counterDoc._id, counterDoc);
34 }
35 }
36);
37
38export { getCounter, incrementCounter };
39
Let’s write a test to make sure this component renders the value returned from the query above. Later, we can add more tests, such as ensuring that clicking on the button calls the desired mutation (as seen in the full examples linked in the snippets later in this article).
1import Counter from "./Counter";
2import { render } from "@testing-library/react";
3import { describe, it, expect, vi } from "vitest";
4
5const setup = () => render(<Counter />);
6
7describe("Counter", () => {
8 it("renders the counter", async () => {
9 const { getByText } = setup();
10 expect(getByText("Here's the counter: 0")).not.toBeNull();
11 });
12});
13
But wait! This test doesn’t work yet. Since the Counter component invokes Convex queries and mutations, we need some mechanism to replace our Convex functions with fake implementations. If we try to run this test file without doing so, we’ll get an error with the Convex library complaining that a Convex client has not been provided.
Method 1: Mocking the convex import
This method takes advantage of Vitest’s mock to replace the internal useQuery
and useMutation
implementations with versions that call our fake methods defined in the previous code snippet.
1// See the full file here:
2// https://github.com/get-convex/convex-helpers/blob/main/src/components/Counter.mock.test.tsx
3
4import * as convexReact from "convex/react";
5
6vi.mock("convex/react", async () => {
7 const actual = await vi.importActual<typeof convexReact>("convex/react");
8
9 return {
10 ...actual,
11
12 // Not a typo! useQuery in code calls useQueryGeneric under the hood!
13 useQueryGeneric: (queryName: string, args: Record<string, any>) => {
14 if (queryName !== "counter:getCounter") {
15 throw new Error("Unexpected query call!");
16 }
17 return getCounter(args as any);
18 },
19 useMutationGeneric: (mutationName: string) => {
20 if (mutationName !== "counter:incrementCounter") {
21 throw new Error("Unexpected mutation call!");
22 }
23 return incrementCounterMock;
24 },
25 };
26});
27
This method requires few dependencies, but may not work in test environments that don’t provide functionality for mocking external libraries. Read on for a cleaner solution!
Method 2: Using dependency injection to replace the Convex client
Alternatively, we can use dependency injection (AKA having dependencies be arguments to a function, and passing in a different dependency in test vs. our real codebase) to convince the Convex library it’s connected to a real deployment.
We’ve prepared a version of the ConvexReactClient
for testing purposes. The code and TypeScript bindings for ConvexReactClientFake
can be found here.
1// See the full file here:
2// https://github.com/get-convex/convex-helpers/blob/main/src/components/Counter.injection.test.tsx
3
4// Initialize the Convex mock client
5const fakeClient = new ConvexReactClientFake<API>({
6 queries: {
7 // Replace getCounter with a simple function that returns a global value
8 "counter:getCounter": getCounter,
9 },
10 mutations: {
11 // Replace incrementCounter with a mocked function that can be spied on in tests
12 "counter:incrementCounter": incrementCounterMock,
13 },
14});
15
16// The setup function is different here -- we wrap the Counter in a ConvexProvider
17const setup = () =>
18 render(
19 <ConvexProvider client={fakeClient}>
20 <Counter />
21 </ConvexProvider>
22 );
23
In summary, you can use one of the patterns above to write unit tests for React components that call Convex functions.
Have a use case that isn’t covered here? Reach out to us on the Convex community discord.
Convex is the backend platform with everything you need to build your full-stack AI project. Cloud functions, a database, file storage, scheduling, workflow, vector search, and realtime updates fit together seamlessly.