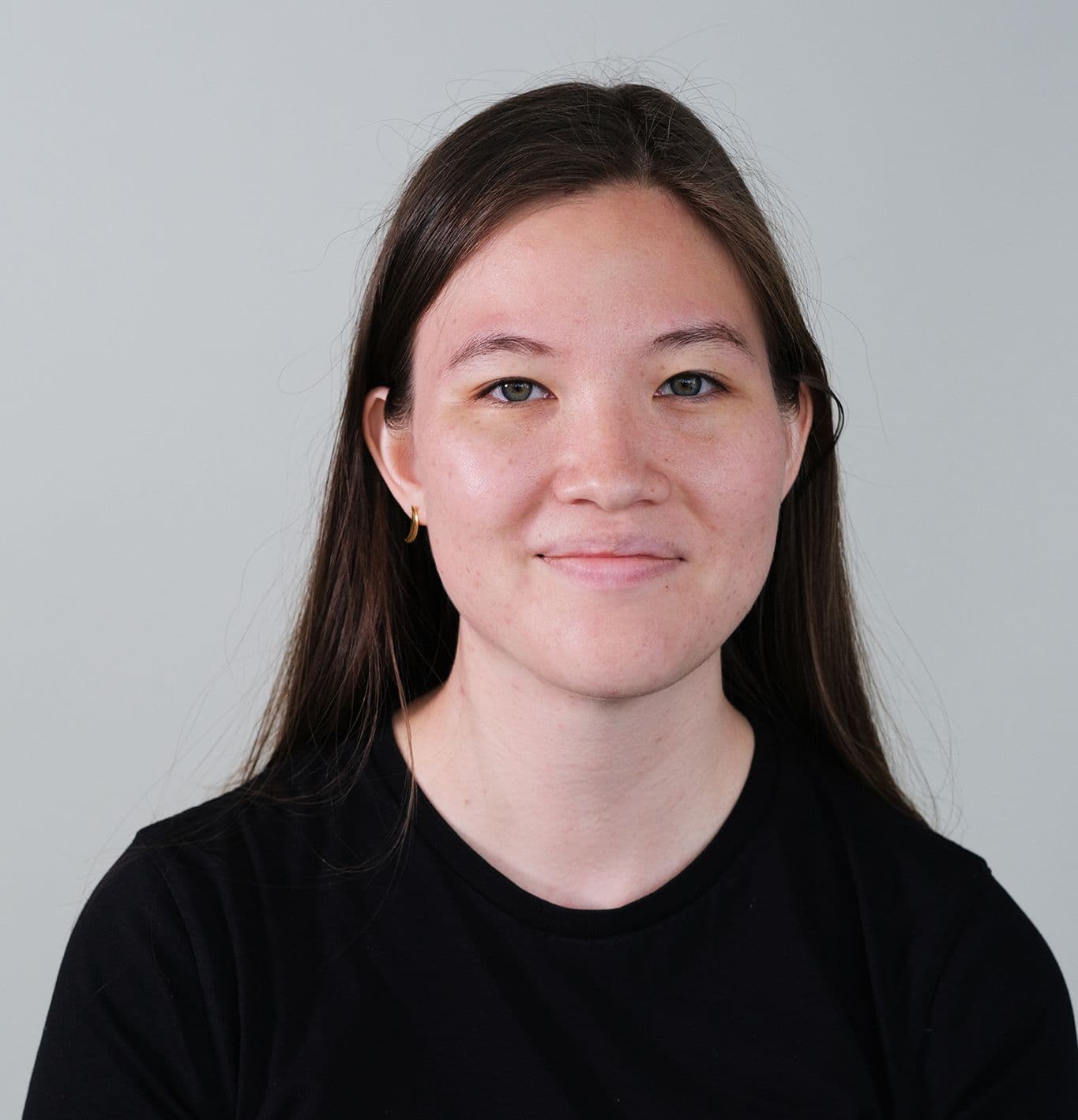
Discord Bot Webhooks with Convex
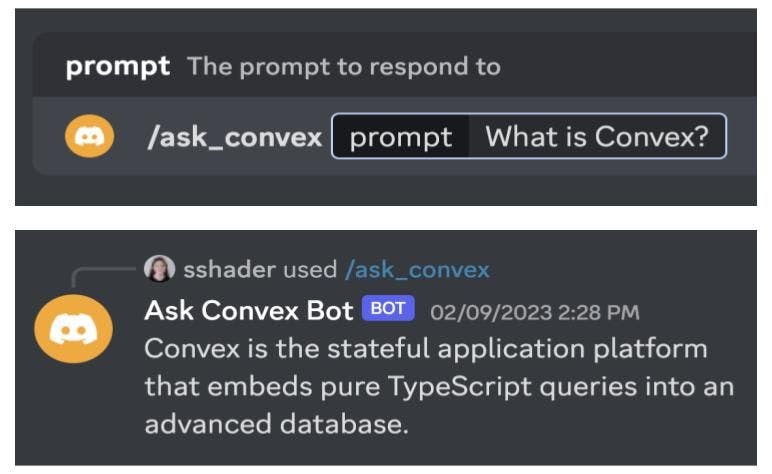
Convex provides client libraries for storing and interacting with data, but sometimes we want a third-party app like Discord to interact with data in Convex. This is often done via a webhook over HTTP, which Convex recently added support for! I used this functionality to build a simple Discord bot that posts custom responses to certain key phrases.
I store my prompts and responses using Convex, and can edit them from a web app using the ConvexReactClient
(shown in orange). When I trigger my Discord bot, Discord sends an HTTP request via a webhook to a configured URL (my Convex deployment), which I handle using an httpAction
(shown in blue).
Diagram
The full code for my app is in https://github.com/sshader/discord-bot, but I'll focus on the webhook specifically.
After setting up my Discord bot and registering a command, I now needed to figure out how to respond to the webhook requests. I can define an endpoint that just returns a successful response to test that everything is hooked up correctly:
1// https://github.com/sshader/discord-bot/blob/stack/convex/http.ts#L9
2const http = httpRouter();
3http.route({
4 path: '/discord',
5 method: 'POST',
6 handler: httpAction(async ({}, request) => {
7 return new Response(null, { status: 200 })
8 })
9});
10
When I try updating my Discord bot settings, I can see in the Convex dashboard that my endpoint is getting run.
Screenshot
Screenshot
Discord requires that you (1) acknowledge a “ping” request and (2) verify the signature of the request and reject it if it’s invalid before it will send your endpoint any webhook events. I used the discord-interactions npm package to verify the signature.
1// https://github.com/sshader/discord-bot/blob/stack/convex/http.ts#L14
2httpAction(async ({}, request) => {
3 const bodyText = await request.text();
4
5 // Check signature -- uses discord-interactions package
6 const isValidSignature = verifyKey(
7 bodyText,
8 request.headers.get('X-Signature-Ed25519'),
9 request.headers.get('X-Signature-Timestamp'),
10 process.env.DISCORD_PUBLIC_KEY
11 )
12 if (!isValidSignature) {
13 return new Response("invalid request signature", { status: 401 });
14 }
15
16 const body = JSON.parse(bodyText);
17 // Handle ping
18 if (body.type === InteractionType.PING) {
19 return new Response(
20 JSON.stringify({ type: InteractionResponseType.PONG }),
21 { status: 200 }
22 )
23 }
24});
25
In addition to the Convex docs it was helpful to look at Cloudflare workers, which have similar JS handlers using the fetch standard. Viewing my endpoint logs in the dashboard helped me catch an issue where I initially did these steps in the reverse order, and so wasn’t verifying signatures on the “ping” requests.
The last part is to query my Convex data and respond to Discord -- I have a Convex query that returns a stores response for a given prompt which I'm using here:
1// https://github.com/sshader/discord-bot/blob/stack/convex/http.ts#L37
2const data = body.data;
3if (
4 body.type === InteractionType.APPLICATION_COMMAND &&
5 data.name === "ask_convex"
6) {
7 const prompt = data.options[0].value;
8 // See https://github.com/sshader/discord-bot/blob/stack/convex/botResponses.ts#L2
9 const botResponse = await runQuery("bot_responses:getBotResponse", { prompt });
10
11 return new Response(
12 JSON.stringify({
13 type: InteractionResponseType.CHANNEL_MESSAGE_WITH_SOURCE,
14 data: { content: botResponse },
15 }),
16 { headers: { "content-type": "application/json" }, status: 200 }
17 );
18}
19
Here’s how it looks all together!
Screenshot of the final app
Convex is the backend platform with everything you need to build your full-stack AI project. Cloud functions, a database, file storage, scheduling, workflow, vector search, and realtime updates fit together seamlessly.