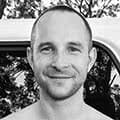
Code Spelunking: Uncovering Convex's API Generation Secrets
I'm the newest member of the Convex team, and as a newcomer, I know I have a lot to learn. One feature that has fascinated me is Convex's automatic API creation, a powerful capability that is one of the reasons why development with Convex feels so fast.
For example I can write a function like this on the server:
1// In "/convex/foo/myQueries.ts"
2
3export const listMessages = query({
4 args: {
5 fromUserId: v.id("users"),
6 },
7 handler: (ctx, args) => {
8 ...
9 },
10});
11
Then on the client I can access this (from React for simplicity) using a really clean, type-safe interface:
1export const UserMessages: React.FC<Props> = ({ fromUserId }) => {
2 const messages = useQuery(api.foo.myQueries.listMessages, { fromUserId });
3 ...
4}
5
I have attempted to write something like this in the past but have never done a great job so I'm super curious to see how they have managed to pull this off.
So join me as I go spelunking through the Convex codebase and work out how this magic works!
Method
Before diving in, I want to mention that there are several ways to approach this kind of investigation.
You could start with the documentation, explore the codebase directly, search online, or ask the system's creators themselves.
I considered taking a shortcut by asking an AI agent like Cursor or Github's Copilot. While that might get me quick answers, I realized this is one of those cases where the journey matters more than the destination. The small insights you gather along the way often prove invaluable when tackling future challenges.
Sometimes asking your all-knowing friend for the answer isn't the best way to learn!
So I decided to go back to first principles and follow the code's breadcrumbs to see where they lead.
Getting Started
Let's start with what we know: when you run the convex dev
command in the terminal, a process generates files in the convex/_generated
directory.
https://github.com/a16z-infra/ai-town/blob/e66da914f0418a202098b01a16b3d5a38cac2997/convex/_generated/api.d.ts
There's a few files here but one one I'm most interested in is api.d.ts
as its the one that seems to be where all that automatic type-safe API magic is coming from.
So I guess a sensible place to start would be to checkout the convex dev
command. Lets start by looking at the npm package for the convex
CLI command and seeing where it points to.
From the referenced source-code we can see that the package.json lists “bin” which is how Node knows what do do when you run a command provided by a package.
1 ...
2 "bin": {
3 "convex": "bin/main-dev",
4 "convex-bundled": "bin/main.js"
5 },
6 ...
7
If we look in the bin directory in the repo and open the main-dev
file we can see that its a simple bash script that does something different depending if its windows or mac.
1#!/bin/bash
2# Run the Convex CLI directly from source code.
3
4if [ "$(uname)" == "Darwin" ] || [ "$(expr substr $(uname -s) 1 5)" == "Linux" ]; then
5 SCRIPTDIR="$(echo "$0" | python3 -c 'import os; print(os.path.dirname(os.path.realpath(input())))')"
6 CONVEX_RUNNING_LIVE_IN_MONOREPO=1 "exec" "$SCRIPTDIR/../node_modules/.bin/tsx" "$SCRIPTDIR/../src/cli/index.ts" "$@"
7else # it's probably Windows
8 # This doesn't follow symlinks quite as correctly as the Mac/Linux solution above
9 CONVEXDIR="$(dirname "$(dirname "$0")")"
10 CONVEX_RUNNING_LIVE_IN_MONOREPO=1 "exec" "$CONVEXDIR/node_modules/.bin/tsx" "$CONVEXDIR/src/cli/index.ts" "$@"
11fi
12
It seems like no matter the platform you are always going to be running the code defined in the /src/cli/index.ts
file, so lets look there next.
1...
2const program = new Command();
3 program
4 .name("convex")
5 .usage("<command> [options]")
6 .description("Start developing with Convex by running `npx convex dev`.")
7 .addCommand(login, { hidden: true })
8 .addCommand(init, { hidden: true })
9 .addCommand(reinit, { hidden: true })
10 .addCommand(dev) // <-- this is the one we are looking for
11 .addCommand(deploy)
12 .addCommand(deployments, { hidden: true })
13 .addCommand(run)
14 .addCommand(convexImport)
15 .addCommand(dashboard)
16 ....
17
As expected this file lists the main “program” and is using the excellent Commander library to help with the CLI.
Lets just confirm this by typing convex --help
and noting the output:
Cool, lets continue exploring the dev
command specifically which is handily defined in dev.ts
Right at the bottom of the file we have what looks like the main part of the command:
1 promises.push(
2 watchAndPush(
3 ctx,
4 {
5 ...credentials,
6 verbose: !!cmdOptions.verbose,
7 dryRun: false,
8 typecheck: cmdOptions.typecheck,
9 typecheckComponents: !!cmdOptions.typecheckComponents,
10 debug: false,
11 debugBundlePath: cmdOptions.debugBundlePath,
12 codegen: cmdOptions.codegen === "enable",
13 liveComponentSources: !!cmdOptions.liveComponentSources,
14 },
15 cmdOptions,
16 ),
17 );
18
As a side note, Github's reference sidebar has been invaluable in helping me navigate the codebase and track function references:
So digging into thewatchAndPush
function it looks like it contains the main infinite “dev” loop in it:
1 ...
2 while (true) {
3 const start = performance.now();
4 tableNameTriggeringRetry = null;
5 shouldRetryOnDeploymentEnvVarChange = false;
6 const ctx = new WatchContext(cmdOptions.traceEvents);
7 showSpinner(ctx, "Preparing Convex functions...");
8 try {
9 await runPush(ctx, options);
10 const end = performance.now();
11 ...
12
That "Preparing Convex functions..." is a good sign that we are on the right track as this is what you see when you make a change to a Convex file right before the codegen does its thing.
The runPush
function looks to be the next port of call on our journey..
We are now in components.ts
and this function:
1export async function runPush(ctx: Context, options: PushOptions) {
2 const { configPath, projectConfig } = await readProjectConfig(ctx);
3 const convexDir = functionsDir(configPath, projectConfig);
4 const componentRootPath = await findComponentRootPath(ctx, convexDir);
5 if (ctx.fs.exists(componentRootPath)) {
6 await runComponentsPush(ctx, options, configPath, projectConfig);
7 } else {
8 await runNonComponentsPush(ctx, options, configPath, projectConfig);
9 }
10}
11
The word "components" in the code is likely referring to Convex's new Components system. This appears to be an abstraction layer to handle codebases with and without component support.
Rather than diving into the Pull Request history of this file, let's stay focused on our main investigation.
For now, let's follow the runNonComponentsPush
code path and see where it takes us.
1 ...
2 if (!options.codegen) {
3 logMessage(
4 ctx,
5 chalk.gray("Skipping codegen. Remove --codegen=disable to enable."),
6 );
7 // Codegen includes typechecking, so if we're skipping it, run the type
8 // check manually on the query and mutation functions
9 const funcDir = functionsDir(configPath, projectConfig);
10 await typeCheckFunctionsInMode(ctx, options.typecheck, funcDir);
11 } else {
12 await doCodegen(
13 ctx,
14 functionsDir(configPath, projectConfig),
15 options.typecheck,
16 options,
17 );
18 if (verbose) {
19 logMessage(ctx, chalk.green("Codegen finished."));
20 }
21 }
22 ...
23
Hmm this if
block looks promising. As a side note, its interesting that you can turn off codegen, I'm not exactly sure why you would want to but its there regardless 🤷
doCodegen
function sounds like what we are after so lets explore it a bit more
1export async function doCodegen(
2 ctx: Context,
3 functionsDir: string,
4 typeCheckMode: TypeCheckMode,
5 opts?: { dryRun?: boolean; generateCommonJSApi?: boolean; debug?: boolean },
6) {
7 const { projectConfig } = await readProjectConfig(ctx);
8 const codegenDir = await prepareForCodegen(ctx, functionsDir, opts);
9
10 await withTmpDir(async (tmpDir) => {
11 // Write files in dependency order so a watching dev server doesn't
12 // see inconsistent results where a file we write imports from a
13 // file that doesn't exist yet. We'll collect all the paths we write
14 // and then delete any remaining paths at the end.
15 const writtenFiles = [];
16
17 // First, `dataModel.d.ts` imports from the developer's `schema.js` file.
18 const schemaFiles = await doDataModelCodegen(
19 ctx,
20 tmpDir,
21 functionsDir,
22 codegenDir,
23 opts,
24 );
25 writtenFiles.push(...schemaFiles);
26
27 // Next, the `server.d.ts` file imports from `dataModel.d.ts`.
28 const serverFiles = await doServerCodegen(ctx, tmpDir, codegenDir, opts);
29 writtenFiles.push(...serverFiles);
30
31 // The `api.d.ts` file imports from the developer's modules, which then
32 // import from `server.d.ts`. Note that there's a cycle here, since the
33 // developer's modules could also import from the `api.{js,d.ts}` files.
34 const apiFiles = await doApiCodegen(
35 ctx,
36 tmpDir,
37 functionsDir,
38 codegenDir,
39 opts?.generateCommonJSApi || projectConfig.generateCommonJSApi,
40 opts,
41 );
42 writtenFiles.push(...apiFiles);
43
44 // Cleanup any files that weren't written in this run.
45 for (const file of ctx.fs.listDir(codegenDir)) {
46 if (!writtenFiles.includes(file.name)) {
47 recursivelyDelete(ctx, path.join(codegenDir, file.name), opts);
48 }
49 }
50
51 // Generated code is updated, typecheck the query and mutation functions.
52 await typeCheckFunctionsInMode(ctx, typeCheckMode, functionsDir);
53 });
54}
55
Let's examine this function in detail since it has some interesting components and helpful comments that make it easy to follow.
The await withTmpDir(async (tmpDir) =>
helper is particularly elegant—it provides a clean way to handle temporary files that get automatically cleaned up after use. While there's a small risk that temporary files might remain if the CLI crashes during codegen, the operating system should eventually handle cleanup.
The comment about writing files in dependency order is intriguing. Though I'd like to explore this concept further, let's bookmark it for now and move forward.
Among several possible paths to explore, I'm particularly interested in the generation of the api.d.ts
file, so let's investigate the doApiCodegen
function next.
1 ...
2 const absModulePaths = await entryPoints(ctx, functionsDir);
3 const modulePaths = absModulePaths.map((p) => path.relative(functionsDir, p));
4
5 const apiContent = apiCodegen(modulePaths);
6 await writeFormattedFile(
7 ctx,
8 tmpDir,
9 apiContent.JS,
10 "typescript",
11 path.join(codegenDir, "api.js"),
12 opts,
13 );
14 ...
15
Most of this function seems to deal with actually writing the api files out to disk. We may return to this but for now I'm interested in how it works out which files and functions it should export out to the api.d.ts
file.
It looks like entryPoints
might be a good place to head next as the name seems to suggest its responsible for finding the “entry points” into the API.
1export async function entryPoints(
2 ctx: Context,
3 dir: string,
4): Promise<string[]> {
5 const entryPoints = [];
6
7 for (const { isDir, path: fpath, depth } of walkDir(ctx.fs, dir)) {
8 if (isDir) {
9 continue;
10 }
11 const relPath = path.relative(dir, fpath);
12 const parsedPath = path.parse(fpath);
13 const base = parsedPath.base;
14 const extension = parsedPath.ext.toLowerCase();
15 ...
16
So the first thing I see here is the walkDir
function which appears to be a recursive directory walker. This makes sense as convex functions could be nested arbitrarily deep within directories, do you need a way to recursively iterate over this tree structure.
Hmmm.. It seems like most of the ~100 line entryPoints
function deals with logging. There is a little bit at the end that deals with excluding ts files that dont contain export
or input
in them
1 // If using TypeScript, require that at least one line starts with `export` or `import`,
2 // a TypeScript requirement. This prevents confusing type errors described in CX-5067.
3 const nonEmptyEntryPoints = entryPoints.filter((fpath) => {
4 // This check only makes sense for TypeScript files
5 if (!fpath.endsWith(".ts") && !fpath.endsWith(".tsx")) {
6 return true;
7 }
8 const contents = ctx.fs.readUtf8File(fpath);
9 if (/^\s{0,100}(import|export)/m.test(contents)) {
10 return true;
11 }
12 ...
13
This is interesting but its not what was expecting to see. I was expecting some kind of AST parser that works out whether the given file contains Convex functions in it or not and then if it does then it should be included in the API.
Instead what im seeing is that so long as the file includes import
or export
within its not a _deps
, _generated
or http router file then its considered to be a file that has an “entry point” in it.
So what's going on here? How does this code work?
1export const UserMessages: React.FC<Props> = ({ fromUserId }) => {
2 const messages = useQuery(api.foo.myQueries.listMessages, { fromUserId });
3 ...
4}
5
How does Typescript and the JS runtime know that there is a callable Convex function at api.foo.myQueries.listMessages
?
I think at this point it is probably a good idea to take a step back and re-asses how I thought API generation works.
Typescript secrets
Lets take a look at an actual api.d.ts
file more deeply. Using use the one from the excellent AI Town project as an example we see:
1declare const fullApi: ApiFromModules<{
2 "agent/conversation": typeof agent_conversation;
3 "agent/embeddingsCache": typeof agent_embeddingsCache;
4 "agent/memory": typeof agent_memory;
5 "aiTown/agent": typeof aiTown_agent;
6 "aiTown/agentDescription": typeof aiTown_agentDescription;
7 "aiTown/agentInputs": typeof aiTown_agentInputs;
8 "aiTown/agentOperations": typeof aiTown_agentOperations;
9 "aiTown/conversation": typeof aiTown_conversation;
10 "aiTown/conversationMembership": typeof aiTown_conversationMembership;
11 "aiTown/game": typeof aiTown_game;
12 "aiTown/ids": typeof aiTown_ids;
13 "aiTown/inputHandler": typeof aiTown_inputHandler;
14 "aiTown/inputs": typeof aiTown_inputs;
15 "aiTown/insertInput": typeof aiTown_insertInput;
16 "aiTown/location": typeof aiTown_location;
17 "aiTown/main": typeof aiTown_main;
18 "aiTown/movement": typeof aiTown_movement;
19 "aiTown/player": typeof aiTown_player;
20 "aiTown/playerDescription": typeof aiTown_playerDescription;
21 "aiTown/world": typeof aiTown_world;
22 "aiTown/worldMap": typeof aiTown_worldMap;
23 constants: typeof constants;
24 crons: typeof crons;
25 "engine/abstractGame": typeof engine_abstractGame;
26 "engine/historicalObject": typeof engine_historicalObject;
27 http: typeof http;
28 init: typeof init;
29 messages: typeof messages;
30 music: typeof music;
31 testing: typeof testing;
32 "util/FastIntegerCompression": typeof util_FastIntegerCompression;
33 "util/assertNever": typeof util_assertNever;
34 "util/asyncMap": typeof util_asyncMap;
35 "util/compression": typeof util_compression;
36 "util/geometry": typeof util_geometry;
37 "util/isSimpleObject": typeof util_isSimpleObject;
38 "util/llm": typeof util_llm;
39 "util/minheap": typeof util_minheap;
40 "util/object": typeof util_object;
41 "util/sleep": typeof util_sleep;
42 "util/types": typeof util_types;
43 "util/xxhash": typeof util_xxhash;
44 world: typeof world;
45}>;
46export declare const api: FilterApi<
47 typeof fullApi,
48 FunctionReference<any, "public">
49>;
50export declare const internal: FilterApi<
51 typeof fullApi,
52 FunctionReference<any, "internal">
53>;
54
Here we can see all TypeScript modules are combined into one large object type. Interestingly, even modules likeutil/assertNever
are included in this API type, despite containing just a single helper function:
1// From https://www.typescriptlang.org/docs/handbook/unions-and-intersections.html#union-exhaustiveness-checking
2export function assertNever(x: never): never {
3 throw new Error(`Unexpected object: ${JSON.stringify(x)}`);
4}
5
Aha! The magic that determines which files should be included in the client-accessible API happens at the TypeScript level, not during codegen!
This is fascinating. Let's verify this by examining the ApiFromModules
type that fullApi
uses.
1
2/**
3 * Given the types of all modules in the `convex/` directory, construct the type
4 * of `api`.
5 *
6 * `api` is a utility for constructing {@link FunctionReference}s.
7 *
8 * @typeParam AllModules - A type mapping module paths (like `"dir/myModule"`) to
9 * the types of the modules.
10 * @public
11 */
12export type ApiFromModules<AllModules extends Record<string, object>> =
13 FilterApi<
14 ApiFromModulesAllowEmptyNodes<AllModules>,
15 FunctionReference<any, any, any, any>
16 >;
17
Ah, seeing that comment above the type makes things clearer.
Let's dig one type deeper and examine that FilterApi
type
1/**
2 * @public
3 *
4 * Filter a Convex deployment api object for functions which meet criteria,
5 * for example all public queries.
6 */
7export type FilterApi<API, Predicate> = Expand<{
8 [mod in keyof API as API[mod] extends Predicate
9 ? mod
10 : API[mod] extends FunctionReference<any, any, any, any>
11 ? never
12 : FilterApi<API[mod], Predicate> extends Record<string, never>
13 ? never
14 : mod]: API[mod] extends Predicate
15 ? API[mod]
16 : FilterApi<API[mod], Predicate>;
17}>;
18
Oof, that is one mind-bending recursive conditional type. After a bit of consultation with buddy ChatGPT I can inform you that what its doing is creating a nice type that reflects our API perfectly. Its going to exclude module exports that aren't Convex functions and is in turn going to exclude modules that contain no function references.
But wait a minute, if this is all just magical Typescript type stuff how are we able to write a chained object at runtime on the client and NOT have it throw a runtime error?
In search of magic
To explain what I mean here, open any webpage in chrome and then open the console (F11) and type this:
1const api = {};
2console.log(api.foo.myQueries.listMessages)
3
You will be greeted by a lovely cannot read properties of undefined
error:
This makes sense right? Because even though JS is quite permissive its not permissive enough to allow you to access properties of an object that have yet to be defined.
So back to the original question, how on earth does Convex let us have that lovely function reference experience where we can define a function reference like api.foo.myQueries.listMessages
if its not the codegen in the CLI that is doing it?
That api
object must be something special 🤔 Lets take a closer look.
Going back to the AI Town example we can see that api.js
in the convex/_generated
directory defines the api
object as anyApi
from the “convex/server” package:
1import { anyApi } from "convex/server";
2
3/**
4 * A utility for referencing Convex functions in your app's API.
5 *
6 * Usage:
7 * ```js
8 * const myFunctionReference = api.myModule.myFunction;
9 * ```
10 */
11export const api = anyApi;
12export const internal = anyApi;
13
If we follow through to where this is defined in the api.ts
in the “get-convex/convex-js” repo we find this export:
1/**
2 * A utility for constructing {@link FunctionReference}s in projects that
3 * are not using code generation.
4 *
5 * You can create a reference to a function like:
6 * ```js
7 * const reference = anyApi.myModule.myFunction;
8 * ```
9 *
10 * This supports accessing any path regardless of what directories and modules
11 * are in your project. All function references are typed as
12 * {@link AnyFunctionReference}.
13 *
14 *
15 * If you're using code generation, use `api` from `convex/_generated/api`
16 * instead. It will be more type-safe and produce better auto-complete
17 * in your editor.
18 *
19 * @public
20 */
21export const anyApi: AnyApi = createApi() as any;
22
My Spidey Sense is tingling I feel like we are getting close.
So what does createApi
do?
1/**
2 * Create a runtime API object that implements {@link AnyApi}.
3 *
4 * This allows accessing any path regardless of what directories, modules,
5 * or functions are defined.
6 *
7 * @param pathParts - The path to the current node in the API.
8 * @returns An {@link AnyApi}
9 * @public
10 */
11function createApi(pathParts: string[] = []): AnyApi {
12 const handler: ProxyHandler<object> = {
13 get(_, prop: string | symbol) {
14 if (typeof prop === "string") {
15 const newParts = [...pathParts, prop];
16 return createApi(newParts);
17 } else if (prop === functionName) {
18 if (pathParts.length < 2) {
19 const found = ["api", ...pathParts].join(".");
20 throw new Error(
21 `API path is expected to be of the form \`api.moduleName.functionName\`. Found: \`${found}\``,
22 );
23 }
24 const path = pathParts.slice(0, -1).join("/");
25 const exportName = pathParts[pathParts.length - 1];
26 if (exportName === "default") {
27 return path;
28 } else {
29 return path + ":" + exportName;
30 }
31 } else if (prop === Symbol.toStringTag) {
32 return "FunctionReference";
33 } else {
34 return undefined;
35 }
36 },
37 };
38
39 return new Proxy({}, handler);
40}
41
I knew it! All JS magic ultimately ends with Proxy Objects.. JavaScript's most magical of magical APIs.
If you aren't familiar with Proxys in JS they basically let you intercept calls to an object be it a get, set, function call and a bunch of other things so that it makes it look like you are using a normal JS objects but no you being tricked, much like being told the world is round when it is quite clearly a torus.
https://imgur.com/gallery/flat-earth-fan-club-AoXqdYG
So what the createApi
function does is create a Proxy object that converts the "dot path" into a string when you use it.
For example, api.something.count
gets transformed into "api/something:count"
.
This makes perfect sense when you think about it, the client needs to convert these function references into API calls to send to the server. It needs to use the same format as Convex's REST API.
In fact the documentation has a section where it explains exactly this:
Client libraries in languages other than JavaScript and TypeScript use strings instead of API objects:
api.myFunctions.myQuery
is"myFunctions:myQuery"
api.foo.myQueries.myQuery
is"foo/myQueries:myQuery"
.api.myFunction.default
is"myFunction:default"
or"myFunction"
.
Super cool!
This is also the syntax you would use if you were going to run a function from the CLI for example: npx convex run api/something:count
Summary
So let's summarize what happens when we run convex dev
:
- Codegen begins by identifying all "entry points". Every entry point gets exported, regardless of whether it contains a Convex function.
- The
ApiFromModules
andFilterApi
types, along with their sub-types, handle the filtering. They remove any exported modules and functions that shouldn't be in the API, leaving us with a clean record type that maps the path to our Convex functions. - The
api
object receives this type, but at runtime it's actually a Proxy object. This enables the client library to convert paths likeapi.foo.myQueries.myQuery
into strings like"foo/myQueries:myQuery"
Where to from here?
Now that we have a better understanding of how things work under the covers, where can we go from here? Here are some ideas I've been thinking about:
- Exclusion list - It would be cool if we could specify a list of paths in the Convex config that should be excluded from the generated API. This would improve TypeScript performance and allow users to maintain an older API on the server while gently discouraging clients from using it, since the TypeScript type wouldn't include the excluded functions.
- Custom TypeScript plugin - did you know you could write plugins for the TypeScript compiler? I know right, super cool. I can imagine a plugin that leverages all this learning to help with function refactors.
- Function redirects - This idea is similar to the exclusion list, but instead of totally excluding a function, we could redirect it or have other functions redirect to it. This would help with API migrations for long-lived projects.
Since this post is getting quite long, these ideas will have to wait for next time. Stay tuned and ping me a message on the Convex Discord to let me know which one you think I should tackle first!
Convex is the backend platform with everything you need to build your full-stack AI project. Cloud functions, a database, file storage, scheduling, workflow, vector search, and realtime updates fit together seamlessly.