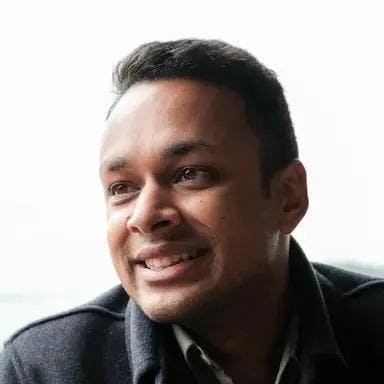
Who's on Call? Learn to Sync Pagerduty with Slack
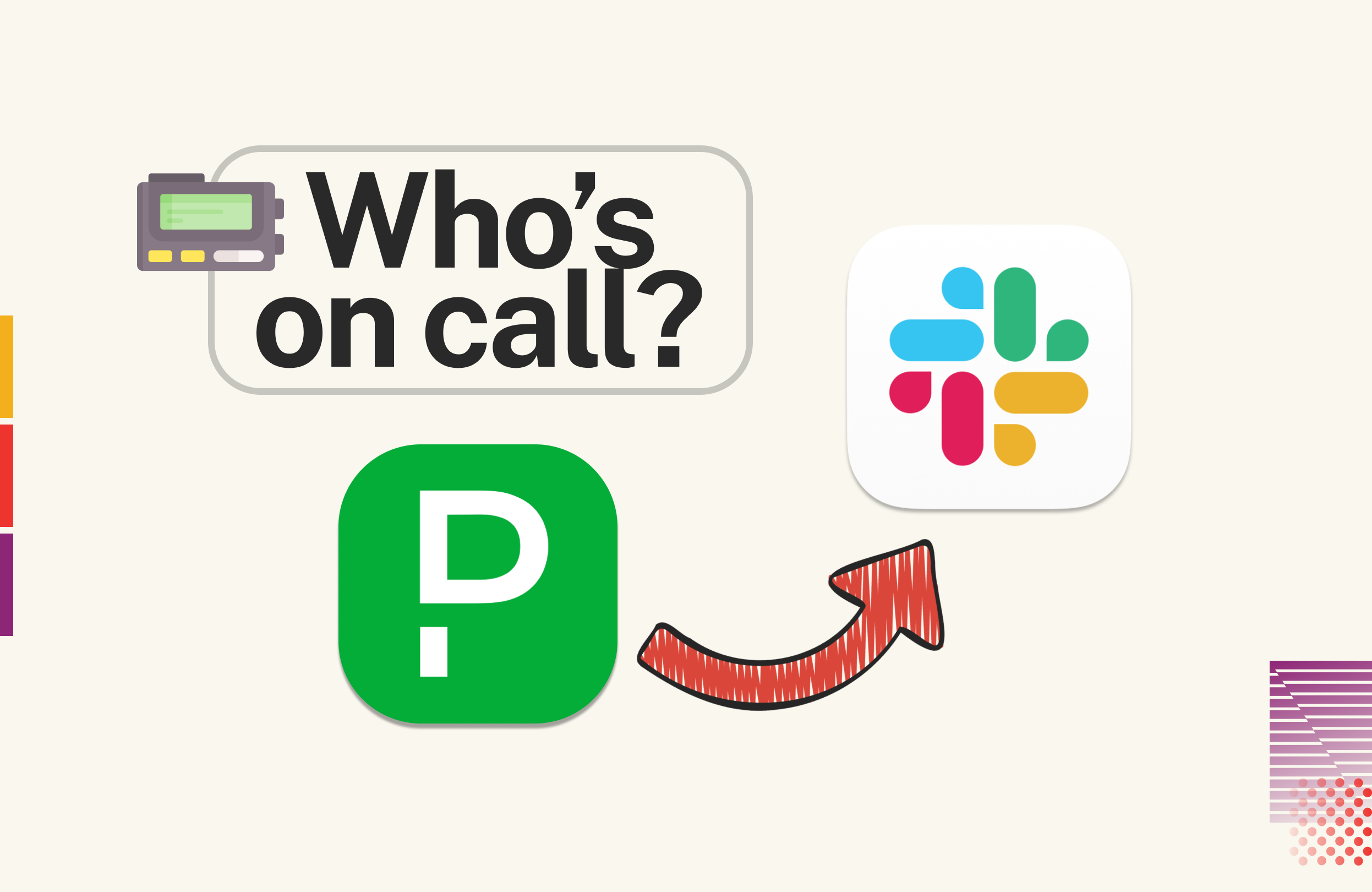
If you use PagerDuty and Slack you could probably benefit from automatically changing the topic for an #oncall Slack channel to display who’s currently on call. You can get this running in a manner of minutes using this repo.
All you need to do is clone the repository, deploy the application to Convex, then enter your API keys and configuration entries.
1git clone https://github.com/get-convex/pagerduty-slack-sync.git
2cd pagerduty-slack-sync
3npm install
4npx convex deploy
5# Set up the PagerDuty and Slack API keys on the dashboard. See README.md for details.
6npx convex dashboard
7
And that’s it!
Read on to see how it’s built.
Sync PagerDuty to Slack in minutes with Convex
At Convex, we use PagerDuty to handle our alerting needs so engineers can keep Convex running smoothly. PagerDuty has a powerful interface for creating multiple schedules letting engineers add temporary overrides, but this means that in the heat of an incident, it can be hard to quickly figure out who is actually on-call.
PagerDuty’s scheduling interface
Ideally, we wanted to see the current primary and secondary on-call engineer at a glance in Slack — where most incidents are discussed and resolved. While PagerDuty doesn’t have a built-in integration for this, we found this repository from the folks at PagerDuty which sets up a function in AWS Lambda to update your Slack channel topic with the current on-call status. It looked perfect for our needs — we followed the instructions to set up the CloudFormation deployment in AWS, set up the API Keys in AWS Systems Manager Parameter Store, stored the configuration in AWS DynamoDB, and a few hours later had PagerDuty updates streaming into our Slack channel.
Architecture diagram from the PagerDuty repository
Wait. A few hours? Surely we can do better than that!
The task at hand sounds simple enough: on a schedule, check who is on-call via the PagerDuty API. Send a request via the Slack API to update the channel topic with the latest state. This is a perfect fit for Cron Job Scheduled Functions on Convex!
We can re-use the ideas from the original PagerDuty repository and write simple Convex functions to accomplish our task.
Let’s start with a function to fetch the current on-call user for a given PagerDuty schedule:
getOncallUser
1// convex/sync.ts
2
3async function getOncallUser(schedule: string): Promise<string> {
4 const token = process.env.PAGERDUTY_API_KEY;
5 if (token === undefined || token == "") {
6 throw "PagerDuty API Key not set";
7 }
8 const headers = {
9 Accept: "application/vnd.pagerduty+json;version=2",
10 Authorization: `Token token=${token}`,
11 };
12
13 const normal_url = `https://api.pagerduty.com/schedules/${schedule}/users`;
14 const override_url = `https://api.pagerduty.com/schedules/${schedule}/overrides`;
15 const now = new Date();
16 let since = new Date(now);
17 // The PagerDuty API gets all users on-call for a schedule within a time bound. The easiest way to get the current on-call is to set a tight bound.
18 since.setSeconds(since.getSeconds() - 5);
19 let payload: Record<string, string> = {};
20 payload["since"] = since.toISOString();
21 payload["until"] = now.toISOString();
22 let parameters = new URLSearchParams(payload);
23 let query_string = `?${parameters.toString()}`;
24
25 let normal_schedule = await fetch(normal_url + query_string, {
26 headers: headers,
27 });
28 if (normal_schedule.status == 404) {
29 throw `Invalid schedule ${schedule}`;
30 }
31 const response = await normal_schedule.json();
32 const users = response["users"];
33 const user = users[0];
34 if (!user) {
35 return "No one :panic:";
36 }
37 let username = user["name"];
38 if (!username) {
39 return "Deactivated user :panic:";
40 }
41 let override_schedule = await fetch(override_url + query_string, {
42 headers: headers,
43 });
44 if ((await override_schedule.json())["overrides"].length > 0) {
45 username += " (Override)";
46 }
47 return username;
48}
49
We’ve chosen to store the PagerDuty API key in an environment variable so it doesn’t need to be committed to your repository.
Similarly, we’ll need to write a function that can set the topic of a given Slack channel. For extra style points, we’ll first get the current topic and preserve its contents when setting a new on-call user.
getSlackTopic
and updateSlackTopic
1// convex/sync.ts
2
3async function getSlackTopic(channel: string): Promise<string> {
4 const token = process.env.SLACK_API_KEY;
5 if (token === undefined || token == "") {
6 throw "Slack API Key not set";
7 }
8 const payload = {
9 token: token,
10 channel: channel,
11 };
12 let response = await fetch("https://slack.com/api/conversations.info", {
13 method: "POST",
14 body: new URLSearchParams(payload).toString(),
15 headers: {
16 "Content-Type": "application/x-www-form-urlencoded; charset=utf-8",
17 },
18 }).then((r) => r.json());
19 try {
20 const topic = response["channel"]["topic"]["value"];
21 return topic;
22 } catch (e) {
23 console.error(e);
24 console.info(response);
25 throw `Could not find channel ${channel} on Slack; ensure the bot is in this channel`;
26 }
27}
28
29const updateSlackTopic = async function (
30 channel: string,
31 proposed_update: string
32): Promise<void> {
33 const token = process.env.SLACK_API_KEY;
34 if (token === undefined || token == "") {
35 throw "Slack API Key not set";
36 }
37 let payload: Record<string, string> = {
38 token: token,
39 channel: channel,
40 };
41
42 let current_topic = await getSlackTopic(channel);
43 if (current_topic == "") {
44 current_topic = ".";
45 }
46 const pipe = current_topic.indexOf("|");
47 if (pipe !== undefined) {
48 let oncall_message = current_topic.substring(0, pipe).trimEnd();
49 current_topic = current_topic.substring(pipe + 1).trimStart();
50 if (oncall_message == proposed_update) {
51 console.log("No topic update required");
52 return;
53 }
54 }
55 proposed_update = proposed_update + " | " + current_topic;
56 payload["topic"] = proposed_update;
57 if (process.env.DRY_RUN !== undefined) {
58 console.log("Would update topic with request", payload);
59 console.log("Exiting without sending request");
60 return;
61 }
62 const response = await fetch("https://slack.com/api/conversations.setTopic", {
63 method: "POST",
64 headers: {
65 "Content-Type": "application/x-www-form-urlencoded; charset=utf-8",
66 },
67 body: new URLSearchParams(payload).toString(),
68 });
69 if (response.status != 200) {
70 const error = await response.text();
71 throw `Failed to update topic: ${error}`;
72 }
73};
74
Note that we also added a dry-run mode for testing without actually updating the topic.
Next, we need a way to store our configuration such that it’s easy to add and remove Slack channels and PagerDuty schedules. Turns out Convex is pretty good at storing documents!
Let’s write a simple schema for our configuration documents:
1// convex/schema.ts
2import { defineSchema, defineTable } from "convex/server";
3import { v } from "convex/values";
4
5export default defineSchema({
6 // Each `config` defines a list of PagerDuty schedules (with names)
7 // to fetch, and a Slack channel ID to update.
8 configs: defineTable({
9 channel: v.string(),
10 schedules: v.array(v.object({ schedule: v.string(), name: v.string() })),
11 }),
12});
13
Lastly, we can wrap this all up in an Action that iterates over the configs and performs the updates, then set up a cron job to call our function every minute.
Action and Cron
1// convex/sync.ts
2export const getConfig = internalQuery({
3 handler: async (ctx) => {
4 return await ctx.db.query("configs").collect();
5 },
6});
7
8async function performUpdate(config: Doc<"configs">) {
9 console.log("Updating for config:", config);
10 let topic = "";
11 for (const schedule of config.schedules) {
12 const oncall = await getOncallUser(schedule.schedule);
13 if (topic != "") {
14 topic += ", ";
15 }
16 topic += `${schedule.name}: ${oncall}`;
17 }
18 await updateSlackTopic(config.channel, topic);
19}
20
21export default action({
22 handler: async (ctx) => {
23 const configs = await ctx.runQuery(internal.sync.getConfig);
24 await Promise.all(configs.map(performUpdate));
25 },
26});
27
28// convex/crons.ts
29import { cronJobs } from "convex/server";
30import { api } from "./_generated/api";
31
32const crons = cronJobs();
33crons.interval("Update Slack topic", {minutes: 1}, api.sync.default);
34
35export default crons;
36
You can find the completed code for this integration here.
Sync PagerDuty to Slack in minutes with Convex
And that’s it — deploying it is as simple as cloning this repository, setting up your Convex project, and configuring your API keys. You can add or remove configurations from the Convex dashboard and you’ll see changes immediately reflect in the linked Slack channel. No advanced degrees in AWS services required!
Convex is the backend platform with everything you need to build your full-stack AI project. Cloud functions, a database, file storage, scheduling, workflow, vector search, and realtime updates fit together seamlessly.