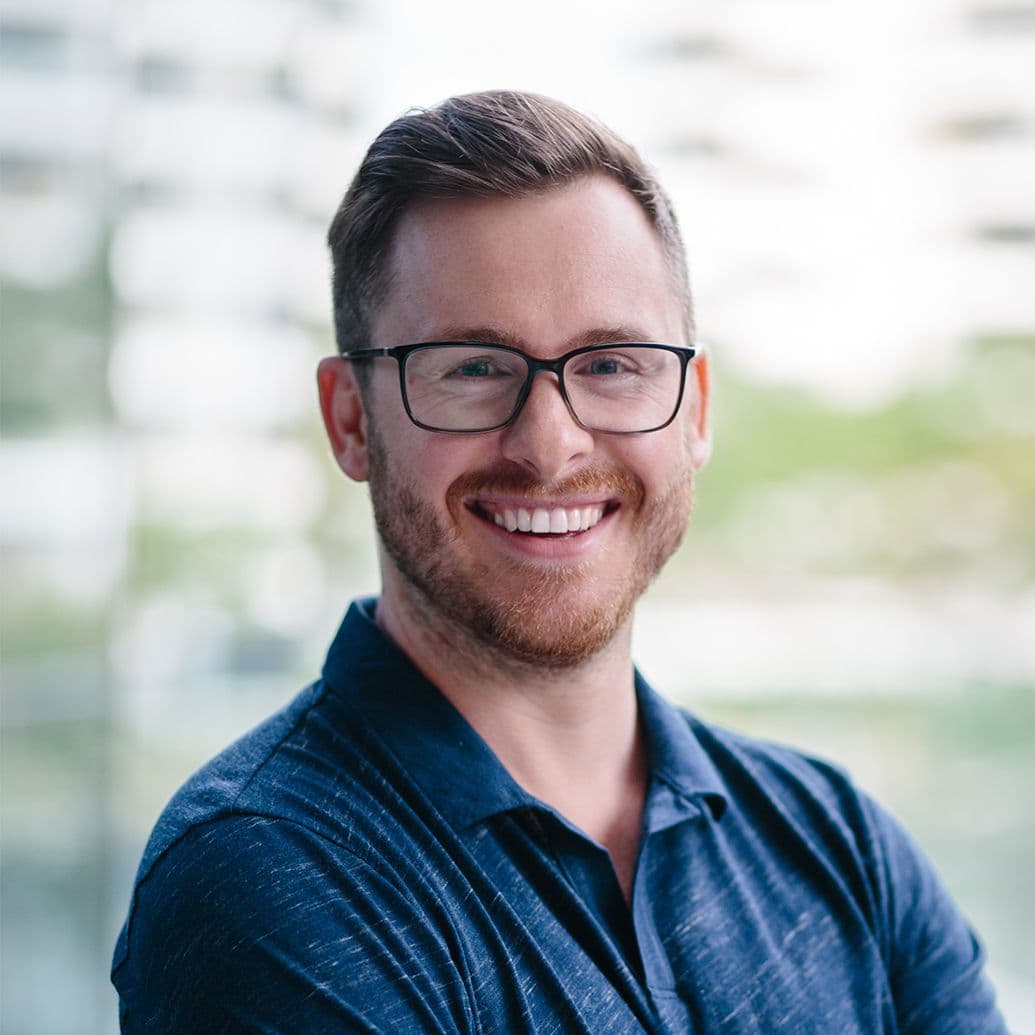
Using Cursor, Claude and Convex to Build a Social Media Scheduling App
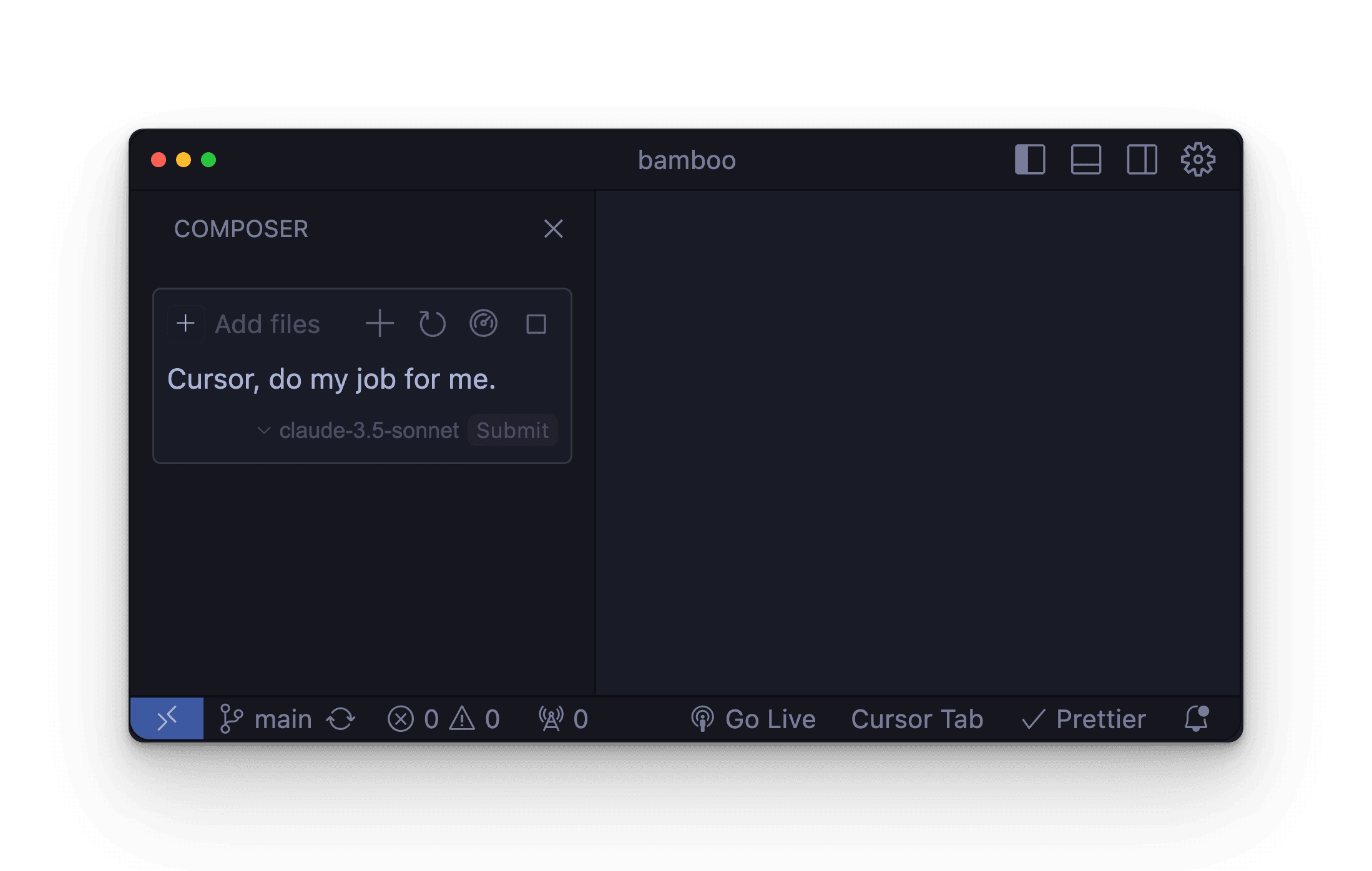
Recently I gave myself a challenge.
I wanted to see if I could prompt my way through developing a complete full stack application using the shiny new tools available to all us: Cursor, Claude (Sonnet 3.5), and Convex. Affectionately known as the c3 stack, if only in my head.
To give myself the best chance of success, I chose to build something I already knew well: a social media scheduling application.
Functionally it's pretty straight forward. The app should be able to connect multiple social accounts, pull in historical posts and metrics, and create new posts be published at some time in the future.
To do this with AI, my mental map went something like this:
- prompt Cursor to scaffold an app with the stack of my choice
- progressively layer in lower-level functionality like social connections
- then layer on higher-order features, like creating a post and display analytics
Prompting Cursor to Scaffold my App: FAIL
This was my first hurdle.
It became immediately apparent that I would not be able to prompt my way through the entire process.
While the tooling we have is undeniably powerful, it's not yet capable of completing most nontrivial tasks without varying degrees of hand-holding.
First things first, I asked Cursor to scaffold my app:
1We're going to develop a social media scheduling application together.
2Please set up a new project using Vite, React, Convex, Tailwind and Shadcn.
3
Cursor & Claude were able to produce something of a scaffolded app, however it never really stuck to the stack I had requested, nor did it use the correct commands or packages.
On the first attempt, it scaffolded a Next.js app, and then layered in (some of) the other integrations. On subsequent attempts, it created what seemed like all the permutations of the stacks I didn't ask for. But it was trying and damnit that's gotta count for something.
In all seriousness, I didn't hold this against Cursor or Claude (to the extend you can hold something against a robot who's trying help). First of all, this was the most trivial part of getting the basics of a new app up and running.
Normally, Claude and Cursor handle triviality with ease. The difference here however is the surface area involved. Trivial yes, because we have doc after doc about how to setup and configure any stack you want. But it's the surface area involved in this step that I believe was causing the two C's to trip up.
Asking Claude to cover too much ground in a single request is a receipe for trouble.
Remember that even the best and brightest LLMs have limited context windows. Not only that, but only a subset their context is "perfectly recallable". For Claude 3.5, this is between 90-95%, depending on the context length provided (source). For reference, Claude 3.5 has a context length of 200k tokens.
Make no mistake, this is very impressive. However, in the – ahem – context of programming applications, you could quickly use up 200k tokens and still be missing context.
I'm speculating, but my hunch is that Cursor is being clever about the context it's sending during requests, attempting to include all of the relevant context to the LLM without overburdening it with non-relevant information. The result is that Claude has good knowledge but not perfect knowledge. And therein lays the surface area problem.
Larger surface areas result in spottier context for Claude who will, in all fairness, do their darndest to complete the task at hand. But as their handler, it's better for us to set them up for success by providing relatively limited-scope requests.
Moving On: Building the UI with Cursor & Claude
I accepted that I'll just have to scaffold the app myself the way I want it. No problem.
1$ npm create convex@latest -- -t react-vite-shadcn
2$ cd my-app
3$ npm i
4$ npm run dev
5
Bingo bango bongo, we're off to the races!
With my app up and running, I wanted to drop in a full UI that I could then adapt for my use case. Typically, you'd want to design your own app, particularly if you're building a real product and making real product choices! For my purposes, effectively research, product design wasn't my primary concern.
So I grabbed the Mail app example from shadcn and I used that as my base:
At this point, a lot in my codebase has changed and I wanted to make sure Cursor was aware. So I asked it to reindex my codebase before moving forward. This feature can be found in Cursor Settings > Features > Codebase indexing.
I'm not sure how often I should do it, or even if it's necessary. But the feature is there and it gives me peace of mind, so shrug emoji.
Let's take stock of where we are at this stage:
- I've scaffolded my app with the stack of my choice
- I've plopped in a pre-existing app UI, courtesy of shadcn
- I've asked Cursor to reindex my codebase
Now I'm ready to ask it for changes. And boy, did it deliver!
1Hi Claude, hope you're well. Here you will find a basic web app, built with Vite, React, Convex, and Shadcn. I've used the "Mail" example for the UI, but we need to update that to reflect our app, which is a social media scheduling app.
2
3First, update the left nav so that's it a list of social networks, with icons, and NOT the nav for a mail app. Right now you'll find it has "Inbox", "Drafts", etc. I like the layout of this design, but we need to update it to be suitable for a social media scheduling app.
4
5Let's update that section to be a list of social networks (with their respective icons):
6- Instagram
7- Facebook
8- YouTube
9- X
10- LinkedIn
11
12@codebase
13
Like a charm. With no handling, we get this result:
Feeling confident
With this nice little win under my belt, it felt like it was time to start making moves!
1Great work!
2
3Let's make some updates to the rest of the UI.
4
5Update @mail-list.tsx to represent a list of sent posts from the selected the social network.
6
7The list should include:
8- the post caption
9- the account it was sent from
10- metrics for views and comments
11- the date it was posted
12
This one added the right UI changes, but didn't automatically update our placeholder datasource, so one little reminder was required:
1Can you update @data.tsx to match the structure you just created, and then update the imports in @mail-list.tsx to match.
2
One more error to fix:
1"selectedNetwork" is not found in @mail-list.tsx
2
Lo and behold, by golly it worked!
Ok! Let's update the preview to show the post instead of mail.
1Nailed it!
2
3Ok, let's now update @mail-display.tsx to show more details about the selected post. It should show a preview of the post - assume instagram - and the metrics for that posts.
4
5It should update when the selected post in @mail-list.tsx is changed, so we can navigate between our posts.
6
Beep beep boop. Apply All.
Oops, one little error to fix:
I could fix this, it's pretty straight forward. But let's use Claude:
1Getting this error:
2
3Uncaught SyntaxError: The requested module '/src/components/mail-display.tsx?t=1727704835419' does not provide an export named 'PostDisplay' (at mail.tsx:32:10)
4
At this point, Cursor & Claude has said it updated the file, and provided the code, but the file itself did not have the changes.
1The changes didn't apply. Please try again - update @mail-display.tsx
2
I tried a few ways, but ultimately had to copy and paste the changes myself.
With a little coddling, we got what we wanted:
At this point, we're about an hour in to developing our app and we have it up and running, with a UI blocked in well enough to get going with the real functionality.
Setting up your Facebook Developer Application
I have some experience with the Facebook Graph API, and so I had a good idea on how I wanted to implement the functionality. This was important background for me to be able to provide Cursor & Claude with specific steps for implementation.
When building something like this for Facebook, there are a number of steps to go through to use the Graph API.
-
Create a Meta developer account
-
Create a new Facebook App
- When prompted for type of app, choose "Other"
- When prompted for type of app, choose "Other"
-
Add 'Facebook Login for Business' as a product, create a new configuration, and select the required permissions for your use case.
- Note: before you can make your app live, you will need to go through Facebook App Review and provide (a) specific justification for every permission and (b) a screen capture of the relevant feature in action
The Facebook developer dashboard can be tricky to navigate, but most of that is out of scope for this article. Reach out to me directly if you have any questions about navigating this particular gauntlet!
The short of it is this: to continue, we need a Facebook App ID, secret, and configuration ID with the requisite permissions. With your Facebook app in Dev mode, we can carry on.
Using Cursor to Develop with Convex
Now that we have our Facebook app setup, we can put it to use. By creating a link, you can kick off the Facebook OAuth flow:
1const facebookLoginUrl = `https://www.facebook.com/20.0/dialog/oauth?client_id=<YOUR_APP_ID>&redirect_uri=https://localhost:5173&scope=email&response_type=code&state=<WHATEVER_YOU_WANT>&auth_type=rerequest&config_id=<YOUR_CONFIG_ID>`;
2
This will launch the Facebook login dialog and because we have specified auth_type=rerequest
, it will always prompt the user to sign-in. Having set the response_type=code
parameter means that on success, Facebook will redirect back to our app to the URI specified with redirect_uri
with a code
param in the URL. This code is short-lived and we must exchange it for a long-lived access token.
From a functional perspective, when our app loads with a code
param in the URL, we need to attempt to exchange it for an access token that we'll use for further requests:
Enter, Cursor:
1If the app is loaded and has a "code" search parameter in the URL, it implies that we have received an auth code from Facebook, that we need to exchange for a Facebook access token.
2
3Let's create this functionality using Convex.
4
5First, check for the code param.
6
7If it exists, create a Convex action that exchanges the code for a token, and stores that token in the Convex database.
8
Huzzah! Cursor has modified our schema.ts
file to support our tokens:
1// ./convex/schema.ts
2
3import { defineSchema, defineTable } from "convex/server";
4import { v } from "convex/values";
5
6// The schema is entirely optional.
7// You can delete this file (schema.ts) and the
8// app will continue to work.
9// The schema provides more precise TypeScript types.
10export default defineSchema({
11 facebookTokens: defineTable({
12 accessToken: v.string(),
13 createdAt: v.number(),
14 }),
15});
16
17
Not only that, but it's created a new Convex action for us to use!
1// ./convex/facebook.ts
2
3import { v } from "convex/values";
4import { action } from "./_generated/server";
5
6export const exchangeCodeForToken = action({
7 args: { code: v.string() },
8 handler: async (ctx, args) => {
9 const { code } = args;
10
11 // Facebook App credentials (store these securely, preferably as environment variables)
12 const clientId = process.env.FACEBOOK_CLIENT_ID;
13 const clientSecret = process.env.FACEBOOK_CLIENT_SECRET;
14 const redirectUri = process.env.FACEBOOK_REDIRECT_URI;
15
16 if (!clientId || !clientSecret || !redirectUri) {
17 throw new Error("Facebook App credentials are not properly configured");
18 }
19
20 // Exchange code for token
21 const response = await fetch(
22 `https://graph.facebook.com/v12.0/oauth/access_token?client_id=${clientId}&redirect_uri=${redirectUri}&client_secret=${clientSecret}&code=${code}`,
23 { method: "GET" },
24 );
25
26 if (!response.ok) {
27 throw new Error("Failed to exchange code for token");
28 }
29
30 const data = await response.json();
31 const accessToken = data.access_token;
32
33 // Store the access token in the database
34 await ctx.db.insert("facebookTokens", {
35 accessToken,
36 createdAt: new Date(),
37 });
38
39 return { success: true };
40 },
41});
42
43
Ok, this is decent, but it will not work as-is.
First thing that stands out is the Facebook API version is very old. v12.0 is from 2021. So, let's update that first. Hopefully Claude isn't using an API that will have breaking changes.
1// Exchange code for token
2 const response = await fetch(
3 `https://graph.facebook.com/v20.0/oauth/access_token?client_id=${clientId}&redirect_uri=${redirectUri}&client_secret=${clientSecret}&code=${code}`,
4 { method: "GET" },
5 );
6
The rest of this line looks correct.
Worth noting here that it's my preexisting knowledge of the Graph API that allows me to spot what's wrong, if anything.
There is another issue: Convex db
is not available on ctx
object in an action
. We need to have it run a mutation instead:
1//Cursor composer chat
2
3"db" is not available on a `ctx` object from within an Convex action, we need to create a Convex mutation to make that db write, and use "ctx.runMutation" to run the function.
4
5@facebook.ts
6
Cursor managed to fix most of the issue with this prompt:
1// ./convex/facebook.ts
2
3import { v } from "convex/values";
4import { action, mutation } from "./_generated/server";
5
6export const storeFacebookToken = mutation({
7 args: { accessToken: v.string() },
8 handler: async (ctx, args) => {
9 const { accessToken } = args;
10 await ctx.db.insert("facebookTokens", {
11 accessToken,
12 createdAt: new Date().getTime(),
13 });
14 },
15});
16
17export const exchangeCodeForToken = action({
18 args: { code: v.string() },
19 handler: async (ctx, args) => {
20 const { code } = args;
21
22 // Facebook App credentials (store these securely, preferably as environment variables)
23 const clientId = process.env.FACEBOOK_CLIENT_ID;
24 const clientSecret = process.env.FACEBOOK_CLIENT_SECRET;
25 const redirectUri = process.env.FACEBOOK_REDIRECT_URI;
26
27 if (!clientId || !clientSecret || !redirectUri) {
28 throw new Error("Facebook App credentials are not properly configured");
29 }
30
31 // Exchange code for token
32 const response = await fetch(
33 `https://graph.facebook.com/v12.0/oauth/access_token?client_id=${clientId}&redirect_uri=${redirectUri}&client_secret=${clientSecret}&code=${code}`,
34 { method: "GET" },
35 );
36
37 if (!response.ok) {
38 throw new Error("Failed to exchange code for token");
39 }
40
41 const data = await response.json();
42 const accessToken = data.access_token;
43
44 // Store the access token in the database using the mutation
45 await ctx.runMutation(storeFacebookToken, { accessToken });
46
47 return { success: true };
48 },
49});
50
51
However, there is still an issue with this line:
1await ctx.runMutation(storeFacebookToken, { accessToken });
2
It needs to import the generated Convex api from ./convex/_generated/api
:
1import { api } from "./_generated/api";
2...
3await ctx.runMutation(api.facebook.storeFacebookToken, { accessToken });
4
Finally, let's speed run collecting all the necessary data from Facebook:
-
Once we have the access token, we request the user from the Graph API endpoint
/me
1https://graph.facebook.com/v20.0/me?access_token=<TOKEN> 2
-
From the response, we use the
id
to get owned accounts:/v20.0/<FB_ME_ID>/accounts/
-
From that response, we use each account
id
to get any associated Instagram Business Accounts:/v20.0/<ACCOUNT_ID>?fields=instagram_business_account
-
From that response, we use the Instagram business account ID to fetch the data for each Instagram account specifying the fields that we want:
1export const igProfileFields: string[] = [ 2 "id", 3 "name", 4 "ig_id", 5 "website", 6 "username", 7 "biography", 8 "media_count", 9 "follows_count", 10 "followers_count", 11 "profile_picture_url", 12]; 13const profile = await axios.get(`https://graph.facebook.com/v20.0/${igBusinessAccountId}`, { 14 params: { fields: igProfileFields.join(",") }, 15}); 16
At this point, we can save the resulting profile to Convex. I've asked Cursor for help:
1Once we have the token, we need to request some data from Facebook: 2 3We should request: 4'/me', then use that id to get accounts, then for each account, get associated instagram business accounts, then for each of those, get their profile (id, name, website, username, media_count, etc.) 5 6Then let's save that profile to Convex. 7 8Remember, from within a Convex action , to save to Convex you need to use a mutation. To query, you need to use a query. 9 10@Codebase 11
Reviewing the changes to
schema.ts
, this looks correct:1// ./convex/schema.ts 2 3import { defineSchema, defineTable } from "convex/server"; 4import { v } from "convex/values"; 5 6export default defineSchema({ 7 facebookTokens: defineTable({ 8 accessToken: v.string(), 9 createdAt: v.number(), 10 }), 11 instagramProfiles: defineTable({ 12 id: v.string(), 13 name: v.string(), 14 website: v.optional(v.string()), 15 username: v.string(), 16 mediaCount: v.number(), 17 }), 18}); 19
How did it fare when it comes to saving this data to Convex? My first positive signal is this little note in the terminal:
1✔️ 11:48:47 Convex functions ready! (4.02s) 2
This typically indicates there are no syntax errors. Yay!
Here's the resulting
convex/facebook.ts
file - it looks good functionally (sans error handling):1import { v } from "convex/values"; 2import { action, mutation, query } from "./_generated/server"; 3import { api } from "./_generated/api"; 4 5export const storeFacebookToken = mutation({ 6 args: { accessToken: v.string() }, 7 handler: async (ctx, args) => { 8 const { accessToken } = args; 9 await ctx.db.insert("facebookTokens", { 10 accessToken, 11 createdAt: new Date().getTime(), 12 }); 13 }, 14}); 15 16export const storeInstagramProfile = mutation({ 17 args: { 18 id: v.string(), 19 name: v.string(), 20 website: v.optional(v.string()), 21 username: v.string(), 22 mediaCount: v.number(), 23 }, 24 handler: async (ctx, args) => { 25 await ctx.db.insert("instagramProfiles", args); 26 }, 27}); 28 29export const getFacebookToken = query({ 30 args: {}, 31 handler: async (ctx) => { 32 const token = await ctx.db.query("facebookTokens").order("desc").first(); 33 return token?.accessToken; 34 }, 35}); 36 37export const exchangeCodeForToken = action({ 38 args: { code: v.string() }, 39 handler: async (ctx, args) => { 40 const { code } = args; 41 42 // Facebook App credentials (store these securely, preferably as environment variables) 43 const clientId = process.env.FACEBOOK_CLIENT_ID; 44 const clientSecret = process.env.FACEBOOK_CLIENT_SECRET; 45 const redirectUri = process.env.FACEBOOK_REDIRECT_URI; 46 47 if (!clientId || !clientSecret || !redirectUri) { 48 throw new Error("Facebook App credentials are not properly configured"); 49 } 50 51 // Exchange code for token 52 const response = await fetch( 53 `https://graph.facebook.com/v12.0/oauth/access_token?client_id=${clientId}&redirect_uri=${redirectUri}&client_secret=${clientSecret}&code=${code}`, 54 { method: "GET" }, 55 ); 56 57 if (!response.ok) { 58 throw new Error("Failed to exchange code for token"); 59 } 60 61 const data = await response.json(); 62 const accessToken = data.access_token; 63 64 // Store the access token in the database using the mutation 65 await ctx.runMutation(api.facebook.storeFacebookToken, { accessToken }); 66 67 // Fetch user data 68 const meResponse = await fetch( 69 `https://graph.facebook.com/v12.0/me?access_token=${accessToken}`, 70 ); 71 const meData = await meResponse.json(); 72 73 // Fetch accounts 74 const accountsResponse = await fetch( 75 `https://graph.facebook.com/v12.0/${meData.id}/accounts?access_token=${accessToken}`, 76 ); 77 const accountsData = await accountsResponse.json(); 78 79 // For each account, get Instagram business accounts 80 for (const account of accountsData.data) { 81 const igAccountResponse = await fetch( 82 `https://graph.facebook.com/v12.0/${account.id}?fields=instagram_business_account&access_token=${accessToken}`, 83 ); 84 const igAccountData = await igAccountResponse.json(); 85 86 if (igAccountData.instagram_business_account) { 87 const igId = igAccountData.instagram_business_account.id; 88 // Fetch Instagram profile 89 const igProfileResponse = await fetch( 90 `https://graph.facebook.com/v12.0/${igId}?fields=id,name,website,username,media_count&access_token=${accessToken}`, 91 ); 92 const igProfile = await igProfileResponse.json(); 93 94 // Store Instagram profile in Convex 95 await ctx.runMutation(api.facebook.storeInstagramProfile, { 96 id: igProfile.id, 97 name: igProfile.name, 98 website: igProfile.website, 99 username: igProfile.username, 100 mediaCount: igProfile.media_count, 101 }); 102 } 103 } 104 105 return { success: true }; 106 }, 107}); 108 109
We see a few of the same issues though, like the old API version. This is why we need to review everything that is generated. Even though we've previously made these corrections, it still repeats the same mistakes.
Anyway, let's move on and fetch and save the media for displaying in our app:
1Great! 2 3Now, let's fetch the media for each instagram page and save that to a new table in the database. 4
Let's review the
schema.ts
changes:1import { defineSchema, defineTable } from "convex/server"; 2import { v } from "convex/values"; 3 4export default defineSchema({ 5 facebookTokens: defineTable({ 6 accessToken: v.string(), 7 createdAt: v.number(), 8 }), 9 instagramProfiles: defineTable({ 10 id: v.string(), 11 name: v.string(), 12 website: v.optional(v.string()), 13 username: v.string(), 14 mediaCount: v.number(), 15 }), 16 instagramMedia: defineTable({ 17 instagramProfileId: v.string(), 18 id: v.string(), 19 media_type: v.string(), 20 media_url: v.string(), 21 caption: v.optional(v.string()), 22 timestamp: v.string(), 23 like_count: v.number(), 24 comments_count: v.number(), 25 impressions: v.optional(v.number()), 26 reach: v.optional(v.number()), 27 }), 28}); 29 30
Looks good so far!
And the code?
1import { v } from "convex/values"; 2import { action, mutation, query } from "./_generated/server"; 3import { api } from "./_generated/api"; 4 5export const storeFacebookToken = mutation({ 6 args: { accessToken: v.string() }, 7 handler: async (ctx, args) => { 8 const { accessToken } = args; 9 await ctx.db.insert("facebookTokens", { 10 accessToken, 11 createdAt: new Date().getTime(), 12 }); 13 }, 14}); 15 16export const storeInstagramProfile = mutation({ 17 args: { 18 id: v.string(), 19 name: v.string(), 20 website: v.optional(v.string()), 21 username: v.string(), 22 mediaCount: v.number(), 23 }, 24 handler: async (ctx, args) => { 25 await ctx.db.insert("instagramProfiles", args); 26 }, 27}); 28 29export const getFacebookToken = query({ 30 args: {}, 31 handler: async (ctx) => { 32 const token = await ctx.db.query("facebookTokens").order("desc").first(); 33 return token?.accessToken; 34 }, 35}); 36 37export const storeInstagramMedia = mutation({ 38 args: { 39 instagramProfileId: v.string(), 40 media: v.array( 41 v.object({ 42 id: v.string(), 43 media_type: v.string(), 44 media_url: v.string(), 45 caption: v.optional(v.string()), 46 timestamp: v.string(), 47 like_count: v.number(), 48 comments_count: v.number(), 49 impressions: v.optional(v.number()), 50 reach: v.optional(v.number()), 51 }), 52 ), 53 }, 54 handler: async (ctx, args) => { 55 const { instagramProfileId, media } = args; 56 for (const item of media) { 57 await ctx.db.insert("instagramMedia", { 58 ...item, 59 instagramProfileId, 60 }); 61 } 62 }, 63}); 64 65export const exchangeCodeForToken = action({ 66 args: { code: v.string() }, 67 handler: async (ctx, args) => { 68 const { code } = args; 69 70 // Facebook App credentials (store these securely, preferably as environment variables) 71 const clientId = process.env.FACEBOOK_CLIENT_ID; 72 const clientSecret = process.env.FACEBOOK_CLIENT_SECRET; 73 const redirectUri = process.env.FACEBOOK_REDIRECT_URI; 74 75 if (!clientId || !clientSecret || !redirectUri) { 76 throw new Error("Facebook App credentials are not properly configured"); 77 } 78 79 // Exchange code for token 80 const response = await fetch( 81 `https://graph.facebook.com/v20.0/oauth/access_token?client_id=${clientId}&redirect_uri=${redirectUri}&client_secret=${clientSecret}&code=${code}`, 82 { method: "GET" }, 83 ); 84 85 if (!response.ok) { 86 throw new Error("Failed to exchange code for token"); 87 } 88 89 const data = await response.json(); 90 const accessToken = data.access_token; 91 92 // Store the access token in the database using the mutation 93 await ctx.runMutation(api.facebook.storeFacebookToken, { accessToken }); 94 95 // Fetch user data 96 const meResponse = await fetch( 97 `https://graph.facebook.com/v20.0/me?access_token=${accessToken}`, 98 ); 99 const meData = await meResponse.json(); 100 101 // Fetch accounts 102 const accountsResponse = await fetch( 103 `https://graph.facebook.com/v20.0/${meData.id}/accounts?access_token=${accessToken}`, 104 ); 105 const accountsData = await accountsResponse.json(); 106 107 // For each account, get Instagram business accounts 108 for (const account of accountsData.data) { 109 const igAccountResponse = await fetch( 110 `https://graph.facebook.com/v20.0/${account.id}?fields=instagram_business_account&access_token=${accessToken}`, 111 ); 112 const igAccountData = await igAccountResponse.json(); 113 114 if (igAccountData.instagram_business_account) { 115 const igId = igAccountData.instagram_business_account.id; 116 // Fetch Instagram profile 117 const igProfileResponse = await fetch( 118 `https://graph.facebook.com/v20.0/${igId}?fields=id,name,website,username,media_count&access_token=${accessToken}`, 119 ); 120 const igProfile = await igProfileResponse.json(); 121 122 // Store Instagram profile in Convex 123 await ctx.runMutation(api.facebook.storeInstagramProfile, { 124 id: igProfile.id, 125 name: igProfile.name, 126 website: igProfile.website, 127 username: igProfile.username, 128 mediaCount: igProfile.media_count, 129 }); 130 131 // Fetch Instagram media 132 const mediaResponse = await fetch( 133 `https://graph.facebook.com/v20.0/${igId}/media?fields=id,media_type,media_url,caption,timestamp,like_count,comments_count,insights.metric(impressions,reach)&access_token=${accessToken}`, 134 ); 135 const mediaData = await mediaResponse.json(); 136 137 const formattedMedia = mediaData.data.map((item: any) => ({ 138 id: item.id, 139 media_type: item.media_type, 140 media_url: item.media_url, 141 caption: item.caption, 142 timestamp: item.timestamp, 143 like_count: item.like_count, 144 comments_count: item.comments_count, 145 impressions: item.insights?.data[0]?.values[0]?.value, 146 reach: item.insights?.data[1]?.values[0]?.value, 147 })); 148 149 // Store Instagram media in Convex 150 await ctx.runMutation(api.facebook.storeInstagramMedia, { 151 instagramProfileId: igProfile.id, 152 media: formattedMedia, 153 }); 154 } 155 } 156 157 return { success: true }; 158 }, 159}); 160
Amazing! It's improving and NOT repeating some mistakes! For example, its use of
api.
is correct. It's also sitcking to api version 20.0.This is next level stuff.
Using Cursor to tie together our Convex backend with our React frontend
Ok, we have enough to start attaching a few wires. We have a blocked out frontend, and a backend that's handling the data.
Let's connect to our data and show some real posts in the frontend:
1Please update @post-list.tsx and @post-display.tsx to show real posts from the Convex database using a Convex client query. Review @Convex docs to see how to do it.
2
3@Codebase
4
(I took this opportunity to rename some of the files away from "mail" and towards "post".)
This worked beautifully. Claude & Cursor created a new function in facebook.ts
for to use as a query from the client:
1// ./convex/facebook.ts
2
3export const getInstagramMedia = query({
4 args: {},
5 handler: async (ctx) => {
6 const media = await ctx.db
7 .query("instagramMedia")
8 .collect();
9
10 return media.map((post) => ({
11 id: post.id,
12 account: post.instagramProfileId, // We'll need to join with instagramProfiles to get the actual account name
13 network: "Instagram",
14 date: post.timestamp,
15 caption: post.caption || "",
16 views: post.impressions || 0,
17 comments: post.comments_count,
18 likes: post.like_count,
19 shares: 0, // Instagram API doesn't provide share count
20 media_url: post.media_url,
21 media_type: post.media_type,
22 }));
23 },
24});
25
And our frontend code for PostList.tsx
looks like this:
1export function PostList({ onSelectPost }: PostListProps) {
2 const posts = useQuery(api.facebook.getInstagramMedia);
3 // do stuff with posts
4
and PostDisplay.tsx
is equally as exciting:
1export function PostDisplay({ postId }: PostDisplayProps) {
2 const posts = useQuery(api.facebook.getInstagramMedia);
3 const post = posts?.find((p) => p.id === postId) || null;
4 // do stuff with post
5
Does it work?
I'm itching to see this in action, but there are a few minor things we need to do before we can try:
- Give Convex the environment variables it needs to interact with the Graph API
- Build up our Facebook OAuth login URL
- ???
- Profit!
Filling in the blanks, our URL would be (yours will have different client and config values):
1https://www.facebook.com/v20.0/dialog/oauth?client_id=2294697257538519&redirect_uri=https://localhost:5173&scope=email&response_type=code&state=501337&auth_type=rerequest&config_id=1050051203383147
2
Great success!
I've added the above URL to a link in our app. Let's see how it works! Here we'll go through the connection flow, and watch Convex work its magic:
What the h*ck just happened?!
Let's recap what we've done.
In about two hours, we stood up a new app. We blocked out a complex UI. We setup a Facebook app. We got our backend to interact with the Facebook Graph API, and used Convex actions, queries and mutations to ingest and store the data.
We then linked up the frontend UI with our backend datasource, using Convex's sync engine to provide live updates to our application as new data rolled in.
Two hours.
Conclusion
The combination of Cursor, Claude and Convex demonstrates a remarkable level of productivity. We built on the shoulders of giants.
However, there are a couple very important points to take away:
- I already knew how to create an app like this, even without Claude. This was extremely helpful along the way, and I'm not sure I'd have known what to fix (or how to fix it) without this prior knowledge.
- My knowledge allowed me to quickly spot issues and provide detailed guidance on how I wanted Claude to work.
- No part of this codebase would be considered production ready! Honestly, it's a mess. It needs a lot of refactoring before you'd ever ship this to real people.
- We DID get a working prototype complete in a few hours! A little handholding, sure, but the dang thing runs. Now it's really off to the races!
The extremely raw code can be found here: https://github.com/tomredman/raw-claude-project
You'll see what I mean when I say this isn't production ready! That said, feel free to clone and submit PRs - bonus points for using Cursor & Claude to improve it!
Convex is the backend platform with everything you need to build your full-stack AI project. Cloud functions, a database, file storage, scheduling, workflow, vector search, and realtime updates fit together seamlessly.